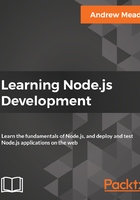
Adding notes to the notes array
The next step in the process of adding notes will be to add the note to the notes array. The notes.push method will let us do just that. The push method on an array lets you pass in an item, which gets added to the end of the array, and in this case, we'll pass in the note object. So we have an empty array, and we add our one item, as shown in the following code; next, we push it in, which means that we have an array with one item:
var addNote = (title, body) => {
var notes = [];
var note = {
title,
body
};
notes.push(note);
};
The next step in the process will be to update the file. Now, we don't have a file in place, but we can load an fs function and start creating the file.
Up above the addNote function, let's load in the fs module. I'll create a const variable called fs and set it equal to the return result from require, and we'll require the fs module, which is a core node module, so there's no need to install it using NPM:
const fs = require('fs');
With this in place, we can take advantage of fs inside the addNote function.
Right after we push our item on to the notes array, we'll call fs.writeFileSync, which we've used before. We know we need to pass in two things: the file name and the content we want to save. For the file, I'll call, notes-data.JSON, and then we'll pass in the content to save, which in this case will be the stringify notes array, which means we can call JSON.stringify passing in notes:
notes.push(note);
fs.writeFileSync('notes-data.json', JSON.stringify(notes));
At this point, when we add a new note, it will update the notes-data.JSON file, which will be created on the machine since it does not exist, and the note will sit inside it. Now, it's important to note that currently every time you add a new note, it will wipe all existing ones because we never load in the existing ones, but we can get started testing that this note works as expected.
I'll save the file, and over inside of Terminal, we can run this file using node app.js. Since we want to add a note, we will be using that add command which we set up, then we'll specify our title and our body. The title flag can get set equal to secret, and for the body flag, I'll set it equal to the Some body here string, as shown here:
node app.js add --title=secret --body="Some body here"
Now, when we run this command from Terminal, we'll see what we'd expect:

As shown in the preceding screenshot, we see a couple of the file commands we added: we see that the add command was executed, and we have our Yargs arguments. The title and body arguments also show up. Inside Atom, we also see that we have a new notes-data.json file, and in the following screenshot, we have our note, with the secret title and the Some body here body:

This is the first step in wiring up that addNote function. We have an existing notes file and we do want to take advantage of these notes. If notes already exist, we don't want to simply wipe them every time someone adds a new note. This means that in notes.js, earlier at the beginning of the addNote function, we'll fetch those notes.