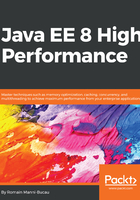
Injections
If you take a look at our quote manager application, you may have noticed that QuoteService was injected in QuoteResource or DirectQuoteSocket. We are exactly in the IoC area of the CDI container. Here, the algorithm globally looks as follows (in pseudo-code):
Object createInstance() {
Object[] constructorArguments = createConstructorArguments(); <1>
Object instance = createNewInstance(constructorArguments); <2>
for each injected field of (instance) { <3>
field.inject(instance);
}
return prepare(instance); <4>
}
To fulfill its role, the CDI will need to instantiate an instance and initialize it. To do so, it proceeds with the following steps which leads to provide you a ready to use instance:
- The CDI allows injections from the constructor parameters, through field injections, or through setter injections. Therefore, before instantiating an instance, the CDI needs to resolve the required parameters and get one instance for each of them.
- Now, the container can provide constructor parameters; it just creates a current instance from the bean constructor.
- Now that the container has an instance, it populates its field/setter injections.
- If needed, the instance is wrapped in a proxy, adding the required services/handlers (interceptors/decorators in CDI semantic).
In terms of the performance, this kind of logic has some consequences for us and the way we can rely on the CDI in high-performance environments and applications. A simple bean instantiation now requires operations which look simple but can be expensive to execute all the time due to the actual work they have to do, like allocating memory or using meta programmation, or because of the complexity they hide:
- Most of the steps imply some reflection (that is, Java reflection) and, therefore, the container must cache all it can to avoid wasting time in retrieving the reflection data again and again.
- Step 1 and step 3 can imply calling back createInstance() for other instances, which means that if the complexity to create an instance without any injection is 1, the complexity to create an instance with N injections will be 1+N. It will be 1+NxM if the N injections have M injections.