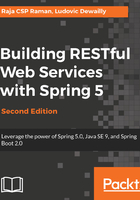
createUser – implementation in the handler and repository
Here, we will define and implement the createUser method in our repository. Also, we will call the createUser method in the main class through UserHandler.
We will add an abstract method for the createUser method in the UserRepository class:
Mono<Void> saveUser(Mono<User> userMono);
Here, we will talk about how to save the user by using the sample repository method.
In UserRepositorySample (the concrete class for UserRepository), we will implement the abstract method createUser:
@Override
public Mono<Void> saveUser(Mono<User> userMono) {
return userMono.doOnNext(user -> {
users.put(user.getUserid(), user);
System.out.format("Saved %s with id %d%n", user, user.getUserid());
}).thenEmpty(Mono.empty());
}
In the preceding code, we used doOnNext to save the user on the repository. Also, the method will return the empty Mono in the case of failure.
As we have added the createUser method in the repository, here we will follow up on our handler:
public Mono<ServerResponse> createUser(ServerRequest request) {
Mono<User> user = request.bodyToMono(User.class);
return ServerResponse.ok().build(this.userRepository.saveUser(user));
}
In the UserHandler class, we have created the createUser method to add a user through a handler. In the method, we extract the request into Mono by the bodyToMono method. Once the user is created, it will be forwarded to UserRepository to save the method.
Finally, we will add the REST API path to save the user in our existing routing function in Server.java:
public RouterFunction<ServerResponse> routingFunction() {
UserRepository repository = new UserRepositorySample();
UserHandler handler = new UserHandler(repository);
return nest (
path("/user"),
nest(
accept(MediaType.ALL),
route(GET("/"), handler::getAllUsers)
)
.andRoute(GET("/{id}"), handler::getUser)
.andRoute(POST("/").and(contentType(APPLICATION_JSON)), handler::createUser)
);
}