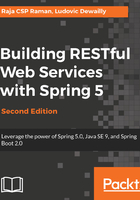
上QQ阅读APP看书,第一时间看更新
deleteUser – implementation in the handler and repository
Here, we will define and implement the deleteUser method in our repository. Also, we will call the deleteUser method in the main class through UserHandler.
As usual, we will add an abstract method for the deleteUser method in the UserRepository class:
Mono<Void> deleteUser(Integer id);
In the UserRepositorySample.java file, we will add the deleteUser method to remove the specified user from the list:
@Override
public Mono<Void> deleteUser(Integer id) {
users.remove(id);
System.out.println("user : "+users);
return Mono.empty();
}
In the preceding method, we simply remove the element from users and return an empty Mono object.
As we have added the deleteUser method in the repository, here we will follow up on our handler:
public Mono<ServerResponse> deleteUser(ServerRequest request) {
int userId = Integer.valueOf(request.pathVariable("id"));
return ServerResponse.ok().build(this.userRepository.deleteUser(userId));
}
Finally, we will add the REST API path to save the user in our existing routing function in Server.java:
public RouterFunction<ServerResponse> routingFunction() {
UserRepository repository = new UserRepositorySample();
UserHandler handler = new UserHandler(repository);
return nest (
path("/user"),
nest(
accept(MediaType.ALL),
route(GET("/"), handler::getAllUsers)
)
.andRoute(GET("/{id}"), handler::getUser)
.andRoute(POST("/").and(contentType(APPLICATION_JSON)), handler::createUser)
.andRoute(PUT("/").and(contentType(APPLICATION_JSON)), handler::updateUser)
.andRoute(DELETE("/{id}"), handler::deleteUser)
);
}