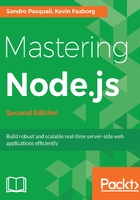
Optimized JavaScript
The JavaScript language is in constant flux, and some major changes and improvements have begun to find their way into native compilers. The V8 engine used in the latest Node builds supports nearly all of the latest features. Surveying all of these is beyond the scope of this chapter. In this section, we'll mention a few of the most useful updates and how they might be used to simplify your code, helping to make it easier to understand and reason about, to maintain, and perhaps even become more performant.
We will be using the latest JavaScript features throughout this book. You can use Promises, Generators, and async/await constructs as of Node 8.x, and we will be using those throughout the book. These concurrency operators will be discussed at depth in Chapter 2, Understanding Asynchronous Event-Driven Programming, but a good takeaway for now is that the callback pattern is losing its dominance, and the Promise pattern in particular is coming to dominate module interfaces.
In fact, a new method util.promisify was recently added to Node's core, which converts a callback-based function to a Promise-based one:
const {promisify} = require('util');
const fs = require('fs');
// Promisification happens here
let readFileAsync = promisify(fs.readFile);
let [executable, absPath, target, ...message] = process.argv;
console.log(message.length ? message.join(' ') : `Running file ${absPath} using binary ${executable}`);
readFileAsync(target, {encoding: 'utf8'})
.then(console.log)
.catch(err => {
let message = err.message;
console.log(`
An error occurred!
Read error: ${message}
`);
});
Being able to easily promisify fs.readFile is very useful.
Did you notice any other new JavaScript constructs possibly unfamiliar to you?