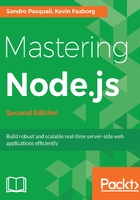
Creating a self-signed certificate for development
In order to support SSL connections, a server will need a properly signed certificate. While developing, it is much easier to simply create a self-signed certificate, which will allow you to use Node's HTTPS module.
These are the steps needed to create a certificate for development. The certificate we create won't demonstrate identity, as a certificate from a third party does, but it is all we need to use the encryption of HTTPS. From a terminal:
openssl genrsa -out server-key.pem 2048
openssl req -new -key server-key.pem -out server-csr.pem
openssl x509 -req -in server-csr.pem -signkey server-key.pem -out server-cert.pem
These keys may now be used to develop HTTPS servers. The contents of these files need simply be passed along as options to a Node server:
const https = require('https');
const fs = require('fs');
https.createServer({
key: fs.readFileSync('server-key.pem'),
cert: fs.readFileSync('server-cert.pem')
}, (req, res) => {
...
}).listen(443);
Free low-assurance SSL certificates are available from http://www.startssl.com/ for cases where self-signed certificates are not ideal during development. Additionally, https://www.letsencrypt.org has started an exciting initiative toward providing free certificates for all (and a safer web).