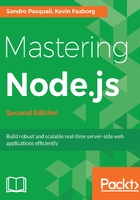
Working with headers
Each HTTP request made to a Node server will likely contain useful header information, and clients normally expect to receive similar package information from a server. Node provides straightforward interfaces for reading and writing headers. We'll briefly go over those simple interfaces, clarifying some details. Finally, we'll discuss how more advanced header usage might be implemented in Node, studying some common network responsibilities a Node server will likely need to accommodate.
A typical request header will look something like the following:

Headers are simple key/value pairs. Request keys are always lowercased. You may use any case format when setting response keys.
Reading headers is straightforward. Read header information by examining the request.header object, which is a 1:1 mapping of the header's key/value pairs. To fetch the accept header from the previous example, simply read request.headers.accept.
The number of incoming headers can be limited by setting the maxHeadersCount property of your HTTP server.
If it is preferred that headers are read programmatically, Node provides the response.getHeader method, accepting the header key as its first argument.
While request headers are simple key/value pairs, when writing headers, we need a more expressive interface. As a response typically must send a status code, Node provides a straightforward way to prepare a response status line and header group in one command:
response.writeHead(200, {
'Content-Length': 4096,
'Content-Type': 'text/plain'
});
To set headers inpidually, you can use response.setHeader, passing two arguments: the header key, followed by the header value.
To set multiple headers with the same name, you may pass an array to response.setHeader:
response.setHeader("Set-Cookie", ["session:12345", "language=en"]);
Occasionally, it may be necessary to remove a response header after that header has been queued. This is accomplished using response.removeHeader, passing the header name to be removed as an argument.
Headers must be written prior to writing a response. It is an error to write a header after a response has been sent.