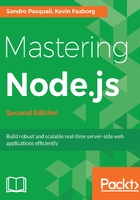
Handling favicon requests
When visiting a URL via a browser, you will often notice a little icon in the browser tab or in the browser's address bar. This icon is an image named favicon.ico, and it is fetched on each request. As such, an HTTP GET request normally combines two requests—one for the favicon, and another for the requested resource.
Node developers are often surprised by this doubled request. Any implementation of an HTTP server must deal with favicon requests. To do so, the server must check the request type and handle it accordingly. The following example demonstrates one method of doing so:
const http = require('http');
http.createServer((request, response) => {
if(request.url === '/favicon.ico') {
response.writeHead(200, {
'Content-Type': 'image/x-icon'
});
return response.end();
}
response.writeHead(200, {
'Content-Type': 'text/plain'
});
response.write('Some requested resource');
response.end();
}).listen(8080);
This code will simply send an empty image stream for the favicon. If there is a favicon to send, you would simply push that data through the response stream, as we've discussed previously.