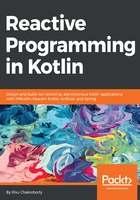
Understanding the Observable.just method
Another interesting factory method is Observable.just; this method creates Observable and adds the parameters passed to it as the only items of the Observable. Note that if you pass an Iterable instance to Observable.just as a single parameter, it will take the entire list as a single item, unlike Observable.from, where it will create items of Observable from each item in Iterable.
Here is what happens when you call Observable.just:
- You call Observable.just with parameters
- Observable.just will create Observable
- It will emit each of its parameters as the onNext notification
- When all parameters are emitted successfully, it will emit the onComplete notification
Let's look at this code example to understand it better:
fun main(args: Array<String>) { val observer: Observer<Any> = object : Observer<Any> { override fun onComplete() { println("All Completed") } override fun onNext(item: Any) { println("Next $item") } override fun onError(e: Throwable) { println("Error Occured ${e.message}") } override fun onSubscribe(d: Disposable) { println("New Subscription ") } }//Create Observer Observable.just("A String").subscribe(observer) Observable.just(54).subscribe(observer) Observable.just(listOf("String 1","String 2","String 3",
"String 4")).subscribe(observer) Observable.just(mapOf(Pair("Key 1","Value 1"),Pair
("Key 2","Value 2"),Pair("Key 3","Value
3"))).subscribe(observer) Observable.just(arrayListOf(1,2,3,4,5,6)).subscribe(observer) Observable.just("String 1","String 2",
"String 3").subscribe(observer)//1 }
And here is the output:

As you can see in the output, lists and maps are also treated as a single item, but look at comment 1 in the code where I passed three strings as parameters of the Observable.just method. Observable.just took each of the parameters as a separate item and emitted them accordingly (see the output).