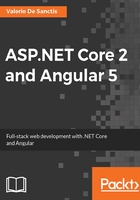
上QQ阅读APP看书,第一时间看更新
AnswerController
Let's go ahead with adding the AnswerController:
using System;
using Microsoft.AspNetCore.Mvc;
using Newtonsoft.Json;
using TestMakerFreeWebApp.ViewModels;
using System.Collections.Generic;
namespace TestMakerFreeWebApp.Controllers
{
[Route("api/[controller]")]
public class AnswerController : Controller
{
// GET api/answer/all
[HttpGet("All/{questionId}")]
public IActionResult All(int questionId)
{
var sampleAnswers = new List<AnswerViewModel>();
// add a first sample answer
sampleAnswers.Add(new AnswerViewModel()
{
Id = 1,
QuestionId = questionId,
Text = "Friends and family",
CreatedDate = DateTime.Now,
LastModifiedDate = DateTime.Now
});
// add a bunch of other sample answers
for (int i = 2; i <= 5; i++)
{
sampleAnswers.Add(new AnswerViewModel()
{
Id = i,
QuestionId = questionId,
Text = String.Format("Sample Answer {0}", i),
CreatedDate = DateTime.Now,
LastModifiedDate = DateTime.Now
});
}
// output the result in JSON format
return new JsonResult(
sampleAnswers,
new JsonSerializerSettings()
{
Formatting = Formatting.Indented
});
}
}
}
As we can see, the code is almost identical to that of QuestionController, although it will most likely change later on.