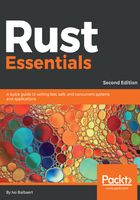
Mutable and immutable variables
Suppose we get a boost from swallowing a health pack and our energy rises to a value of 25. However, if we assign the value to the variable energy as follows:
energy = 25;
We get an error, as follows:
error: re-assignment of immutable variable `energy`.
What is wrong here?
Well, Rust applies programmer's wisdom here: a lot of bugs come from inadvertent or wrong changes of variables, so don't let code change a value unless you have deliberately allowed it!
If you want a mutable variable, because its value can change during code execution, you have to indicate that explicitly with the mut variable, for example:
let mut fuel = 34; fuel = 60;
Simply declaring a variable as let n; is also not enough. We get the following error:
error: type annotations needed, consider giving `energy2` a type, cannot infer type for `_`
Indeed, the compiler needs a value to infer its type.
We can give the compiler this information by assigning a value to the variable n, like n = -2; but as the message says, we could also indicate its type as follows:
let n: i32;
Or:
let n: i32 = -2; // n is a binding of type i32 and value -2
The type (here i32) follows the variable name after a colon : (as we already showed for global constants), optionally followed by an initialization. In general the type is indicated like this-n: T where n is a variable and T a type, and it reads, variable n is of type T. So, this is the inverse of what is done in C or C++, Java or C#, where one would write Tn.
For the primitive types, this can also be done simply with a suffix, as follows:
let x = 42u8; let magic_number = 3.14f64;
Trying to use an uninitialized variable results in the following error:
error: use of possibly uninitialized variable
Local variables have to be initialized before use in order to prevent undefined behavior. When the compiler does not recognize a name (for example, a function name) in your code, you will get the following error:
error: not found in this scope error
It is probably just a typo, but it is caught early on at compilation, and not at runtime!