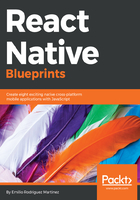
上QQ阅读APP看书,第一时间看更新
Adding state to our screen
Let's add some initial state to our ShoppingList screen to populate the list with actual dynamic data. We will start by creating a constructor and setting the initial state there:
/*** ShoppingList.js ***/
...
constructor(props) {
super(props);
this.state = {
products: [{ id: 1, name: 'bread' }, { id: 2, name: 'eggs' }]
};
}
...
Now, we can render that state inside of <List> (inside the render method):
/*** ShoppingList.js ***/
...
<List>
{
this.state.products.map(p => {
return (
<ListItem
key={p.id}
>
<Body>
<Text style={{ color: p.gotten ? '#bbb' : '#000' }}>
{p.name}
</Text>
</Body>
<Right>
<CheckBox
checked={p.gotten}
/>
</Right>
</ListItem>
);
}
)}
</List>
...
We now rely on a list of products inside our component's state, each product storing an id, a name, and gotten properties. When modifying this state, we will automatically be re-rendering the list.
Now, it's time to add some event handlers, so we can modify the state at the users' command or navigate to the AddProduct screen.