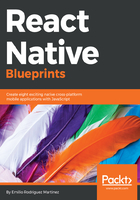
Building the FeedsList screen
The list of feeds will be used as the home screen for this app, so let's focus on building the list of the feeds' titles:
/** * src/screens/FeedsList.js ***/
import React from 'react';
import { Container, Content, List, ListItem, Text } from 'native-base';
export default class FeedsList extends React.Component {
render() {
const { feeds } = this.props.screenProps.store;
return (
<Container>
<Content>
<List>
{feeds &&
feeds.map((f, i) => (
<ListItem key={i}>
<Text>{f.title}</Text>
</ListItem>
))
</List>
</Content>
</Container>
);
}
}
This component expects to receive the list of feeds from this.props.screenProps.store and then iterates over that list building a NativeBase <List>, showing the titles for each of the feeds on the store.
Let's introduce some MobX magic now. As we want our component to be re-rendered when the list of feeds changes (when a feed is added or removed), we have to mark our component with the @observer decorator. MobX will automatically force the component re-rendering on any update. Let's see now how to add the decorator to our component:
...
@observer
export default class FeedsList extends React.Component {
...
That's it. Now, our component will be notified when the store is changed and a re-render will be triggered.