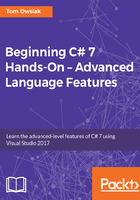
Completing the GenMethods class
For the next stage, you can say the following. Enter it within a set of curly braces below this line:
if(x.CompareTo(y) < 0)
Now, why do we write this? We write this because if you right-click on the CompareTo method and select Go To Definition in the drop-down menu (F12), you can see that it's defined inside the IComparable interface. If you expand that and look at what it returns, it says: Values Meaning Less than zero This instance precedes obj in the sort order., as shown in Figure 2.2.2:

In other words, in our context, this means that x and y are related in the following sense.
If the value returned by CompareTo is less than 0, then x is less than y.
Now, enter the following within a set of curly braces beneath the preceding line:
return $"<br>{x}<{y}";
In this line, you return and actually format a string, so that it's more than just a simple comparison. So, for example, you can say here x is less than y.
The other possibility is the reverse. Now, enter the following beneath the earlier closed curly brace:
else { return $"<br>{x}>{y}"; }
If you want to know more about CompareTo, right-click on it and select Go To Definition in the drop-down menu (F12). As seen under Returns in Figure 7.2.3, it says: Zero This instance occurs in the same position in a sort order as obj. Greater than zero This instance follows object in the sort order.:

In the if (x.CompareTo(y) < 0) line, this instance signifies the x variable and object denotes the y variable.
So, this is the basic GenMethods class. The final version of the GenMethods.cs file, including comments, is shown in the following code block:
using System;
public class GenMethods
{
//method can operate on multiple data types
public static void Swap<T>(ref T x, ref T y)
{
T temp = x; //save x
x = y;//assign y to x
y = temp;//assign original value of x back to y
}
//this function has a constraint, so it can operate on values
//that can be compared
public static string Compare<T>(T x, T y) where T :IComparable
{
//CompareTo returns < 0, =0,or >0, depending on the relationship
//between x and y
if(x.CompareTo(y)<0)
{
return $"<br>{x}<{y}";
}
else
{
return $"<br>{x}>{y}";
}
}
}
As you can see, the GenMethods class contains a couple of generic functions because it can operate on different data types, except for the second CompareTo method, which is a slightly more restricted version, meaning that a constraint is applied of the IComparable type.