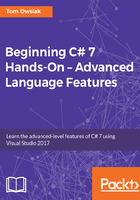
上QQ阅读APP看书,第一时间看更新
Chapter review
For review, the complete version of the Default.aspx.cs file for this chapter, including comments, is shown in the following code block:
using System;//needed for array, Convert, and Converter
delegate double CompareValues(double x, double y);//delegate for defining expression bodied lambda
public partial class _Default :System.Web.UI.Page
{
double FromStringToDouble(string s) => Convert.ToDouble(s);//expression bodied function member
protected void Button1_Click(object sender, EventArgs e)
{
//split entries into array of strings
string[] vals = TextBox1.Text.Split(new char[] { ',' });
//line 10 below converts all strings to doubles using the
//vals array, and a new Converter object
//which really just calls FromStringToDouble
double[] doubleVals =
Array.ConvertAll(vals, new Converter<string, double>(FromStringToDouble));
//lines 13-17 define the expression bodied lambda, this one compares two
//values and returns the bigger
CompareValues compareValues = (xin, yin) =>
{
double x = xin, y = yin;
return x > y ? x : y;
};
//line 19 invokes CompareValuesInList, which needs the lambda, and
//list of values to compare
sampLabel.Text =
CompareValuesInList(compareValues, doubleVals[0], doubleVals[1],doubleVals[2]).ToString();
}
//lines 22-25 below return either third value if it's biggest,
//or one of the other two
static double CompareValuesInList(CompareValues compFirstTwo, double first, double second, double third)
{
return third > compFirstTwo(first, second) ? third : compFirstTwo(first, second);
}
}