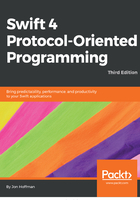
Defining a protocol
The syntax we use to define a protocol is very similar to the syntax used to define a class, structure, or enumeration. The following example shows the syntax used to define a protocol:
Protocol MyProtocol { //protocol definition here }
To define the protocol, we use the protocol keyword followed by the name of the protocol. We then put the requirements, which our protocol defines, between curly brackets. Custom types can state that they conform to a particular protocol by placing the name of the protocol after the type's name, separated by a colon. The following example shows how we would define that a structure conforms to a protocol:
struct MyStruct: MyProtocol { //structure implementation here }
A type can also conform to multiple protocols. We list the multiple protocols that the type conforms to by separating them with commas:
struct MyStruct: MyProtocol, AnotherProtocol, ThirdProtocol { // Structure implementation here }
Having a type conform to multiple protocols is a very important concept within protocol- oriented programming, as we will see later in the chapter and throughout this book. This concept is known as protocol composition.
Now let's see how we would add property requirements to our protocol.