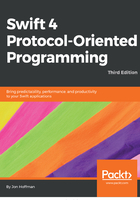
Classes
In object-oriented programming, we cannot create an object without a blueprint that tells the application what properties and methods to expect from the object. In most object- oriented languages, this blueprint comes in the form of a class. A class is a construct that allows us to encapsulate the properties, methods, and initializers of an object into a single type. Classes can also include other items, such as subscripts; however, we are going to focus on the basic items that make up classes not only in Swift, but in other languages as well.
Let's look at how we would use a class in Swift:
class MyClass { var oneProperty: String init(oneProperty: String) { self.oneProperty = oneProperty } func oneFunction() { } }
An instance of a class is typically called an object. However, in Swift, structures and classes have many of the same functionalities; therefore, we will use the term instance when referring to instances of either type.
Anyone who has used object-oriented programming in the past is probably familiar with the class type. It has been the backbone of object-oriented programming since its inception.
When we create instances of the class, it is named; therefore, the class is a named type. The class type is also a reference type.
The next type we are going to look at is arguably the most important type in the Swift language: Structures.