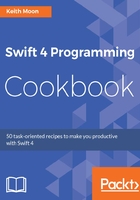
How to do it...
Earlier, we created a Person object to represent people in our model and a PersonName struct to hold information about a person's name. Now, let's turn our attention to a person's title, for example, Mr., Mrs., and so on, which precede someone's full name. There are a small and finite number of common titles that a person may have; therefore, an enum is a great way to model this information.
Enter the following into the playground:
enum Title {
case mr
case mrs
case mister
case miss
case dr
case prof
case other
}
We define our enumeration with the enum keyword and provide a name for the enum. As with classes and structs, the convention is that this starts with a capital letter, and the implementation is defined within curly brackets. We define each enum option with the case keyword, and the convention since Swift 3 is that these start with a lowercase character. Now that we have defined our enum, let's see how to assign it:
let title1 = Title.mr
Enums can be assigned by specifying the enum type, then a dot, and then the case. However, if the compiler can infer the enum type, we can omit the type and just provide the case, preceded by a dot:
let title2: Title
title2 = .mr