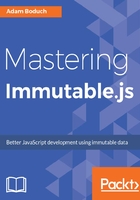
上QQ阅读APP看书,第一时间看更新
Using the clear() method
You don't actually have to create a utility function to empty collections by replacing them with new instances. The clear() method does the same thing:
const myList = List.of(1, 2);
const myMap = Map.of('one', 1, 'two', 2);
// Create new instances by emptying the
// existing instances.
const myEmptyList = myList.clear();
const myEmptyMap = myMap.clear();
console.log('myList', myList.toJS());
// -> myList [ 1, 2 ]
console.log('myEmptyList', myEmptyList.toJS());
// -> myEmptyList []
console.log('myMap', myMap.toJS());
// -> myMap { one: 1, two: 2 }
console.log('myEmptyMap', myEmptyMap.toJS());
// -> myEmptyMap {}
The benefit with the clear() method is that you don't have to invent a utility function that returns new collection instances based on type. Another advantage is that the clear() method will first check to see if the collection is already empty and, if so, it will return the current collection.
Immutable.js does something neat when it creates new collections. The first time an empty collection is created, let's say a list, that same list instance is reused whenever an empty list is needed. If you create an empty list ( List()), or clear a list ( myList.clear()), the same instance is reused every time. This is only possible because collections are immutable.