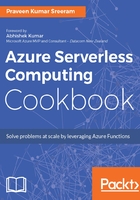
上QQ阅读APP看书,第一时间看更新
How to do it...
- Navigate to the Integrate tab of the RegisterUser function and click on the New Output button to add a new output binding.
- Choose the SendGrid binding and click on the Select button to add the binding.
- Provide the following parameters in the SendGrid output (message) binding:
- Message parameter name: Leave the default value, which is message. We will be using this parameter in the Run method in a moment.
- SendGrid API Key: Provide the App settings key that you have created in Application settings.
- To address: Provide the email address of the administrator.
- From address: Provide the email address from where you would like to send the email. In general, it would be something like donotreply@example.com.
- Message subject: Provide the subject that you would like to have in the email subject.
- Message Text: Provide the email body text that you would like to have in the email body.
- This is how the SendGrid output (message) binding should look like after providing all the fields:

- Once you review the values, click on Save to save the changes.
- Navigate to the Run method and make the following changes:
- Add a new reference for SendGrid and also the namespace SendGrid.Helpers.Mail.
- Add a new out parameter message of type Mail.
- Create an object of type Mail. We will understand how to use this object in the next recipe.
- The following is the complete code of the Run method:
#r "Microsoft.WindowsAzure.Storage"
#r "SendGrid"
using System.Net;
using SendGrid.Helpers.Mail;
using Microsoft.WindowsAzure.Storage.Table;
using Newtonsoft.Json;
public static void Run(HttpRequestMessage req,
TraceWriter log,
CloudTable
objUserProfileTable,
out string
objUserProfileQueueItem,
out Mail message
)
{
var inputs =
req.Content.ReadAsStringAsync().Result;
dynamic inputJson =
JsonConvert.DeserializeObject<dynamic>
(inputs);
string firstname= inputJson.firstname;
string lastname=inputJson.lastname;
string profilePicUrl =
inputJson.ProfilePicUrl;
objUserProfileQueueItem = profilePicUrl;
UserProfile objUserProfile = new
UserProfile(firstname, lastname);
TableOperation objTblOperationInsert =
TableOperation.Insert(objUserProfile);
objUserProfileTable.Execute
(objTblOperationInsert);
message = new Mail();
}
public class UserProfile : TableEntity
{
public UserProfile(string lastName, string
firstname,string profilePicUrl)
{
this.PartitionKey = "p1";
this.RowKey = Guid.NewGuid().ToString();;
this.FirstName = firstName;
this.LastName = lastName;
this.ProfilePicUrl = profilePicUrl;
}
public UserProfile() { }
public string FirstName { get; set; }
public string LastName { get; set; }
public string ProfilePicUrl {get; set;}
}
- Now, let's test the functionality of sending the email by navigating to the RegisterUser function and submitting a request with the some test values, as follows:
{
"firstname": "Bill",
"lastname": "Gates",
"ProfilePicUrl":"https://upload.wikimedia.org/
wikipedia/commons/thumb/1/19/
Bill_Gates_June_2015.jpg/220px-
Bill_Gates_June_2015.jpg"
}