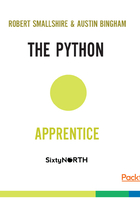
Python return semantics
Python's return statement uses the same pass-by-object-reference semantics as function arguments. When you return an object from a function in Python, what you're really doing is passing an object reference back to the caller. If the caller assigns the return value to a reference, they are doing nothing more than assigning a new reference to the returned object. This uses the exact same semantics and mechanics that we saw with explicit reference assignment and argument passing.
We can demonstrate this by writing a function which simply returns its only argument:
>>> def f(d):
... return d
...
If we create an object such as a list and pass it through this simple function, we see that it returns the very same object that we passed in:
>>> c = [6, 10, 16]
>>> e = f(c)
>>> c is e
True
Remember that is only returns True when two names refer to the exact same objects, so example this shows that no copies of the list were made.