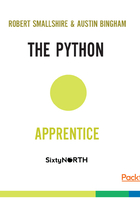
Modifying external objects in a function
To demonstrate Python's argument passing semantics, we'll define a function at the REPL which appends a value to a list and prints the modified list. First we'll create a list and give it the name m:
>>> m = [9, 15, 24]
Then we'll define a function modify() which appends to, and prints, the list passed to it. The function accepts a single formal argument named k:
>>> def modify(k):
... k.append(39)
... print("k =", k)
...
We then call modify(), passing our list m as the actual argument:
>>> modify(m)
k = [9, 15, 24, 39]
This indeed prints the modified list with four elements. But what does our list reference m outside the function now refer to?
>>> m
[9, 15, 24, 39]
The list referred to by m has been modified because it is the self-same list referred to by k inside the function. As we mentioned at the beginning of the section, when we pass an object-reference to a function we're essentially assigning from the actual argument reference, in this case m, to the formal argument reference, in this case k.

As we have seen, assignment causes the assigned-to reference to refer to the same object as the assigned-from reference. This is exactly what's going on here. If you want a function to modify a copy of an object, it's the responsibility of the function to do the copying.