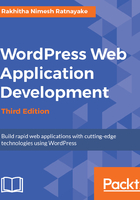
Handling login form submission
We have defined a function call on the init action to call the login_user function. This function is responsible for handling the login form submission and authenticating the users into the application. Let's take a look at the form submission handling part of the login_user function:
public function login_user() {
global $wpwaf_login_params;
$errors = array();
if ( $_POST['wpwaf_login_submit'] ) {
$username = isset ( $_POST['wpwaf_username'] ) ? $_POST['wpwaf_username'] : '';
$password = isset ( $_POST['wpwaf_password'] ) ? $_POST['wpwaf_password'] : '';
if ( empty( $username ) )
array_push( $errors, __('Please enter a username.','wpwaf') );
if ( empty( $password ) )
array_push( $errors, __('Please enter password.','wpwaf') );
if(count($errors) > 0){
include WPWAF_PLUGIN_DIR . 'templates/login-template.php';
exit;
}
$credentials = array();
$credentials['user_login'] = $username;
$credentials['user_login'] = sanitize_user( $credentials['user_login'] );
$credentials['user_password'] = $password;
$credentials['remember'] = false;
// Rest of the code
}
}
As usual, we need to validate the post data and generate the necessary errors to be shown in the frontend. Once validations are successfully completed, we assign all the form data to an array after sanitizing the values. The username and password are contained in the credentials array with the user_login and user_password keys. The remember key defines whether to remember the password or not. Since we don't have a remember checkbox in our form, it will be set to false. Next, we need to execute the WordPress login function in order to log the user into the system, as shown in the following code:
$user = wp_signon( $credentials, false );
if ( is_wp_error( $user ) ){
array_push( $errors, $user->get_error_message() );
$wpwaf_login_params['errors'] = $errors;
}else{
wp_redirect( home_url() );
exit;
}
WordPress handles user authentication through the wp_signon function. We have to pass all the credentials generated in the previous code with an additional second parameter of true or false to define whether to use a secure cookie. We can set it to false for this example. The wp_signon function will return an object of the WP_User or the WP_Error class based on the result.
Once a user is successfully authenticated, a redirection will be made to the home page of the site. Now we should have the ability to authenticate users from the login form in the frontend.