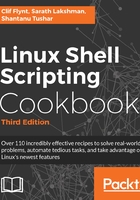
How to do it...
Assign a value to a variable with the equal sign operator:
varName=value
The name of the variable is varName and value is the value to be assigned to it. If value does not contain any space character (such as space), it need not be enclosed in quotes, otherwise it must be enclosed in single or double quotes.
Access the contents of a variable by prefixing the variable name with a dollar sign ($).
var="value" #Assign "value" to var echo $var
You may also use it like this:
echo ${var}
This output will be displayed:
value
Variable values within double quotes can be used with printf, echo, and other shell commands:
#!/bin/bash #Filename :variables.sh fruit=apple count=5 echo "We have $count ${fruit}(s)"
The output will be as follows:
We have 5 apple(s)
Because the shell uses a space to delimit words, we need to add curly braces to let the shell know that the variable name is fruit, not fruit(s).
Environment variables are inherited from the parent processes. For example, HTTP_PROXY is an environment variable that defines which proxy server to use for an Internet connection.
Usually, it is set as follows:
HTTP_PROXY=192.168.1.23:3128 export HTTP_PROXY
The export command declares one or more variables that will be inherited by child tasks. After variables are exported, any application executed from the current shell script, receives this variable. There are many standard environment variables created and used by the shell, and we can export our own variables.
For example, the PATH variable lists the folders, which the shell will search for an application. A typical PATH variable will contain the following:
$ echo $PATH /home/slynux/bin:/usr/local/sbin:/usr/local/bin:/usr/sbin:/usr/bin:/sbin:/bin:/usr/games
Directory paths are delimited by the : character. Usually, $PATH is defined in /etc/environment, /etc/profile or ~/.bashrc.
To add a new path to the PATH environment, use the following command:
export PATH="$PATH:/home/user/bin"
Alternatively, use these commands:
$ PATH="$PATH:/home/user/bin" $ export PATH $ echo $PATH /home/slynux/bin:/usr/local/sbin:/usr/local/bin:/usr/sbin:/usr/bin:/sbin:/bin:/usr/games:/home/user/bin
Here we have added /home/user/bin to PATH.
Some of the well-known environment variables are HOME, PWD, USER, UID, and SHELL.
When using single quotes, variables will not be expanded and will be displayed as it is. This means, $ echo '$var' will display $var.
Whereas, $ echo "$var" will display the value of the $var variable if it is defined, or nothing if it is not defined.