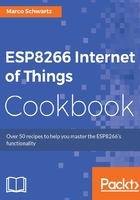
Controlling an LED
This recipe is going to look at how to control an LED using an ESP8266 board. This will basically involve changing the state of an LED either ON or OFF, using the ESP8266 board's GPIO pins. The exercise will enable you to understand how to use the digital output function on the ESP8266.
Getting ready
Connect your ESP8266 board to your computer via a USB cable and set up your Arduino IDE (refer back to Chapter 1, Configuring the ESP8266). Once that is done, you can proceed to make the other connections.
In this recipe, we will need the following components:
- ESP8266 board
- USB cable
- LED (https://www.sparkfun.com/products/528)
- 220 Ω resistor (https://www.sparkfun.com/products/10969)
- Breadboard
- Jumper wires
Start by mounting the LED onto the breadboard. Connect one end of the 220 Ω resistor to the positive leg of the LED (the positive leg of an LED is usually the taller one of the two legs). Connect the other end of the resistor to another rail of the breadboard and connect one end of the jumper wire to that rail and the other end of the jumper wire to pin 5 of the ESP8266 board. Take another jumper wire and connect one of its ends to the negative leg of the LED and connect the other end to the GND pin of the ESP8266. The connection is as shown in the following diagram:

How to do it…
We will use the digitalWrite()
function to output a HIGH
signal on pin 5
for a duration of 1 second and then output a low signal on pin 5
for a duration of one second. This will be repeated over and over again to blink the LED:
// LED pin int ledPin = 5; void setup() { pinMode(ledPin, OUTPUT); } void loop() { // ON digitalWrite(ledPin, HIGH); delay(1000); // OFF digitalWrite(ledPin, LOW); delay(1000); }
- Copy the sketch and paste it in your Arduino IDE.
- Check to ensure that the ESP8266 board is connected. Select the board that you are using in the Tools | Board menu (in this case it is the Adafruit HUZZAH ESP8266).
- Select the serial port your board is connected to from the Tools | Port menu and then upload the code.
How it works…
The program uses the digitalWrite()
function to change the state of the output pin, which in turn changes the state of the LED. Pin 5 is configured as an output in the setup section of the program. In the loop section, pin 5's state is set to HIGH
(digital state 1) for one second. This turns the LED on for one second, after which pin 5's state is set to LOW
(digital state 0) for one second, which turns the LED off for one second.
The digitalWrite()
function is used to control the state of an output pin. The syntax is digitalWrite(pin number, state).
The pin number is the number assigned to the GPIO pin that is being controlled, and the state is the digital output signal (0 or 1). Instead of writing 1 and 0 as the state, we use HIGH
and LOW
respectively.
There's more…
Try to replace the HIGH
and LOW
states with 1 and 0 in the sketch and test it. You can also vary the delays in the sketch to change how the LED blinks.
See also
There is more you can do with LEDs, apart from just turning them on and off. In the next tutorial, we will be looking at how to dim LEDs using the ESP8266.