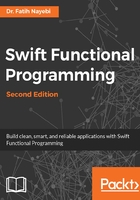
上QQ阅读APP看书,第一时间看更新
Error handling
Swift provides support to throw, catch, propagate, and manipulate recoverable errors at runtime.
Value types should conform to the Error protocol to be represented as errors. The following example presents some 4xx and 5xx HTTP errors as enum:
enum HttpError: Error {
case badRequest
case unauthorized
case forbidden
case requestTimeOut
case unsupportedMediaType
case internalServerError
case notImplemented
case badGateway
case serviceUnavailable
}
We will be able to throw errors using the throw keyword and mark functions that can throw errors with the throws keyword.
We can use a do-catch statement to handle errors by running a block of code. The following example presents JSON parsing error handling in a do-catch statement:
protocol HttpProtocol{
func didRecieveResults(results: Any)
}
struct WebServiceManager {
var delegate:HttpProtocol?
let data: Data
func test() {
do {
let jsonResult = try JSONSerialization.jsonObject(with:
self.data, options: JSONSerialization.ReadingOptions
.mutableContainers)
self.delegate?.didRecieveResults(results: jsonResult)
} catch let error {
print("json error" + error.localizedDescription)
}
}
}
We can use a defer statement to execute a set of statements just before code execution leaves the current code block, regardless of how the execution leaves the current block of code.