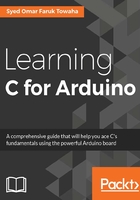
Things to remember
The following sections cover some things you need to remember.
Watch your case
C is case sensitive. So, we need to take care about what we type, or our code won't compile. For example, loop()
and Loop()
are not the same thing in C. We cannot use serial.print("Hello Arduino!");
instead of Serial.print("Hello Arduino!");
.
Don't forget your semicolon!
Now look at the following code. Can you tell where it went wrong?
void setup() { Serial.begin(9600) Serial.println("Arduino is fun"); } void loop() { }
Exactly! On the second line, we missed a semicolon. Let's see if our Arduino IDE can detect the error. We wrote the code in the editor of our Arduino IDE and clicked the verify button (), and found the following result:

From this screen, we can see that our Arduino IDE could detect the error. The log (the black portion of the Arduino IDE) shows where the error occurred. The log says, expected ';' before 'Serial'
, which means we missed a semicolon before Serial.println("Arduino is fun");
.
Adding both Setup() and Loop() functions
You cannot forget to add either the setup()
function or the loop()
function to our code. They must be added to the code. Say we forgot to add the loop()
function. We will see the following error. The same error will occur if we miss the setup()
function:

Minding the baud rate
Say I have added Serial.begin(9600)
to our setup
function. Our code will be as follows:
void setup() { Serial.begin(9600); Serial.println("Today"); Serial.println("is"); Serial.println("a"); Serial.println("wonderful"); Serial.println("day!"); } void loop() { }
Clearly, the output of the code on the serial monitor will be as follows:

But if, on the serial monitor, we accidentally change the baud rate to something else, say, 19200, what will happen? What will the output on the serial monitor be? The output will be similar to the following screenshot:

So, we need to have a look at the baud rate. If you are not doing any high-speed communication keep both the baud rates at 9600.
Formatting your code
Take a look at the following images. Which code is more readable, left or right?

The code on the right is more readable because it clear-coded. So, it is necessary to format our code. We can do it manually. On Arduino IDE, you can format your code automatically, too. To do this automatically, we need to go to Tools
and select Auto Format
. Your code will be formatted as the code on the right-hand side of the preceding image.
Turning the LED On
If you have a closer look at your Arduino Uno, you will see a few LEDs integrated to the board. See the following figure for a better look:

There are four LEDs on the board:
- LED on pin 13 (connected with Arduino pin 13)
- Power LED (turns on when the Arduino is powered)
- Rx and Tx LEDs (turn on when Arduino code is uploaded or data is transferred via 0 and 1 Arduino pins)
We can program the LED connected with pin 13. After we upload any code to our board, usually, the LED on pin 13 turns on.
Now we will write code to control the LED. You can also connect an external LED on pin 13, and a ground pin with a proper resistor, as shown in the following figure. If you have an external LED connected to the board, it will be clear to you if the LED is being controlled by the code, since the integrated LED is small:

Note
The positive leg of the LED will go to pin 13 of the Arduino, and the negative leg will connect to one end of the resistor; the other end of the resistor will go to any of the ground pins on the Arduino. Usually, the longer leg of the LED is positive and the shorter leg is negative. Another way to find out the positive and negative leg is to look through the LED; you will see a flat plate and a sharp plate. The outer part of the sharp plate is positive, and the flat plate's outer leg is negative.
Now let's write our code to turn the LED on. Write the following code in the Arduino text editor, creating a new file on the Arduino Uno:
void setup() { pinMode(13, OUTPUT); } void loop() { }
We know that the setup()
function holds the variables. In the preceding code, we have written a pinMode()
function, where we have passed two parameters: the pin number, and the result of pinMode. pinMode(13, OUTPUT);
means we have selected pin 13. So, we can now control anything connected to pin 13.
The pinMode()
function is for the digital pins of the Arduino board. If you are not sure about the digital pins of the Arduino board, please refer back to Chapter 1, Getting Started
If you want to use any other pins (say, pins 9, 6, 4, and 3), can you guess what you have to write?
Exactly. The pinMode
of the pins will be as follows:
pinMode(9, OUTPUT); pinMode(6, OUTPUT); pinMode(4, OUTPUT); pinMode(3, OUTPUT);
Tip
Be careful to write OUTPUT in all capital letters.
Now we will send a signal to pin 13 to turn the LED on. There are two kinds of signals, LOW and HIGH. LOW is for sending no signal to the pin, and HIGH is for sending a signal to the pin. You can use 0 and 1 instead of LOW and HIGH.
Let's send a HIGH signal to pin 13. Since pin 13 is a digital pin, we need to send the signal using the following function:
digitalWrite(13, HIGH);
The digitalWrite()
function has two parameters; the first one is pin number and the second one is the pulse, or signal. It works for only digital pins. Remember that you cannot use the pin number on this function if you do not write the pinMode()
function with the same pin number. To use a digital pin and give it a signal (either HIGH or LOW), you must write two functions to declare and use the pin. The pinMode()
function is used to declare the pin that we have connected something to, and the digitalWrite()
function allows us to send a signal to the defined pin.
If we want to send no signal to the pin 13, we need to write the following function:
digitalWrite(13, LOW);
Tip
We can use 0 and 1 instead of LOW and HIGH. So, we can write digitalWrite(13, 1)
and digitalWrite(13,
0)
instead of digitalWrite(13, HIGH)
and digitalWrite(13, LOW)
, respectively.
What should we do if we want to turn the LED on? I guess you know that already.
Yes, we need to write the digitalWrite()
function inside the setup()
function, unless we do not want to send the same signal again and again.
So, the code to turn on the LED on pin 13 will be as follows:
void setup() { pinMode(13, OUTPUT); digitalWrite(13, HIGH);//Sends a pulse to pin 13 } void loop() { }
If you upload the code to the board, you will see that the LED is turned on. Let's turn it off now. You can easily guess what the code to turn our LED off will be, right?
It is as follows:
void setup() { pinMode(13, OUTPUT); digitalWrite(13, LOW); //Sends no pulse to pin 13 } void loop() { }
Have you noticed that we can switch our LED on or off from our code without even touching the LED? Isn't it exciting? How about we make it more interesting: let's blink our LED!
Blink a LED
To blink a LED, we need to know about time. I am sure you are asking yourself what should you know about time. Think about blinking a LED. You will see there is a delay between the LED turning and off, right? And it needs to be done repeatedly. Am I thinking the right things? Yes! So, first we need to turn the LED on, then we make a delay, then turn the LED off, and make a delay again. Then repeat the whole thing again. So, what is the algorithm for the blinking LED? Let's see:
- Turn the LED on, SET the pin HIGH.
- Delay sometimes depends on how quickly the blinking will happen.
- Turn the LED off, SET the pin LOW.
- Repeat step 2.
- Repeat steps 1 to 4.
Now you can easily envision how the blinking happens. Let's write our blinking code now. But, before we write the code, let me introduce you to another function, called delay()
. This will handle the waiting between the LED turning on and off. This means this function will stop or hold off on sending signals to the board for a defined time passed inside the function. Inside our delay()
function, we will pass the time in milliseconds. Say we want to wait one second in between turning the LED on and off; we need to write the following:
delay(1000);
Note
1,000 milliseconds = 1 second. So, we need to multiply the time in seconds by 1,000, inside the delay()
function, to get the correct time.
According to our algorithm, the code for blinking will be as follows. We need to write the blinking part of the code inside the loop()
function. Can you guess why? Yes! To make the blinking repeat. The code is as follows:
void setup() { pinMode(13, OUTPUT); digitalWrite(13, HIGH); // you may delete this line, do you know why? } void loop() { digitalWrite(13, HIGH); delay(1000); digitalWrite(13, LOW); delay(1000); }
You can see that we have made pin 13 high, which turns the LED on; then we had a delay of one second and turned the LED off, and again had a delay of one second.
The whole thing runs again and again as we have written the pulse sending part to the pin 13 inside the loop()
function. Let's upload the code and look at the result:

Yes! Our LED is blinking with a one-second delay!
Commenting
Imagine one of your friends knows how to use and programme an Arduino. He wrote some code and e-mailed you. You know a little programming. Let's imagine he e-mailed the following code:

From the code, you can understand only the setup()
and delay()
functions, as we just learned about them a moment ago.
What happens in the rest of the code? Isn't it totally new to you? If your friend had sent you the following code, would not you understand more?

Yes! You can now understand the code a little bit more, right? Why? Because, written in the code is what happens for each of the lines. See that?
In programming, this is known as commenting. It is a good practice to comment on any code that's a little harder for you. You might not remember what you did on a specific line; the commenting will help in that case.
So, how should you write your comment? It is simple. For a single-line comment, you just place two forward slashes before the comment, as follows:
//I am a comment //I will help you to understand the code //I cannot be read by the compiler: D //I am invisible to the compiler: D
For a multi-line comment, you need to follow the following example. All you need to do is place all your long comments inside /*
and */
:
/* I am a comment I will help you to understand the code I cannot be read by the compiler: D I am invisible to the compiler: D */
Let's look at how it will appear in the editor:

You can see that the comments are in gray. The compiler cannot read the comments; only humans can.
Try at home
How about you try the following things at home? Don't worry, they will be explained later:
- Try to understand the code your friend sent you via e-mail.
- Connect an external LED to your Arduino and play with it by changing the values of the
delay()
function. - Upload the code to your board and look at the result. (You might need an external LED for the code-the integrated LED might not work properly.)
Sometimes, we need to work quickly; if you know the keyboard shortcuts, the task becomes much easier.
Keyboard shortcuts
Let's look at a few keyboard shortcuts for Arduino IDE, which will help you to work way faster: