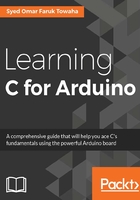
Strings and operations with strings
A string is one of the most important data types in C for Arduino. In this section, we will learn more about string.
By string, we mean a collection of characters. A sentence or word is a string.
In pure C, we don't have the data type String
, but in C for Arduino, we do. Let's look at a basic program using the string data type:
String myString = "Hello, How are you?"; void setup() { Serial.begin(9600); Serial.print(myString); } void loop() { }
The output of the program is as follows:

The output is a plain text that we assigned to our String
variable, myString
. If your string is too long, you can write it as follows:
String myString = " This is the first line" " This is the second line" " This is the third line" " This is the fourth line" " This is the fifth line"; void setup() { Serial.begin(9600); Serial.print(myString); } void loop() { }
The output of the preceding code will be as follows:

Have you noticed that all our output is on one line? Yes, it will be on one single line, even though the string has multiple lines. If we want to use a break after each line, we need to use an escape character (\n
). With the use of \n
, the code's output will be as follows:

Conversion of strings
Other kinds of data types can be converted into a String
data type.
Let's look at few examples.
If you want to convert a character into a String
, you can do the following:
String myString = String('A');
To convert an integer into a String
, you can write the following:
String myString = String(55);
You can convert a string into a String
object too (we will learn more about objects in later chapters):
String myString = String("This String is an object String");
You can convert a decimal number into binary (2-base number: 0 and 1), hexadecimal (16-base number: 0-9 and A-F) or octal (8-base number: 0 to 7), by changing it as follows:
String myString = String(44,BIN); // change decimal to binary String myString = String(44,HEX); //change decimal to hexadecimal String myString = String(44,OCT); //change decimal to octal
If you want to keep a definite decimal point, you can write your float as follows:
String myString = String(44.453534,5); //
. This will show up to five decimal places.
Let's look at the preceding conversation in one code:
String myStringString = String('A'); String myStringInt = String(55); String myStringObject = String("This is a String Object"); String myStringBin = String(44, BIN); String myStringHexa = String(44, HEX); String myStringOcta = String(44, OCT); String myStringDecimal = String(44.453534, 5); void setup() { Serial.begin(9600); Serial.println(myStringString); Serial.println(myStringInt); Serial.println(myStringObject); Serial.println(myStringBin); Serial.println(myStringHexa); Serial.println(myStringOcta); Serial.println(myStringDecimal); } void loop() { }
The output will be as follows:

You can add two strings in a few ways. This process is known as concatenation. Let's assume we have two strings, as follows:
String A = "Hello, Arduino! "; String B = "How are you today? ";
If we want to join the strings together, we can do the following:
String C = A + B;
Now, if we print String C
, we will get the following output:

We can also concatenate Strings
and numbers, as follows:
int numb = 90; String D = "The result is " + numb;
The output will look as follows:

You can replace part of a string with another string. We have the following string:
String A = "Hello, My name is Arduino!";
Now, we want to replace "Hello" with "Hi". We can do this as follows:
String B = A.replace("Hello", "Hi");
Now, the new String B is "Hi, My name is Arduino!";
.
Let's look at the output:

There are lots of operations using strings. We will learn about more operations in later chapters.
Exercise
- Write a program that will print the following outputs (using string operations):
"This is my Lab; it has 2 doors" (use concatenation here and print 2 from another variable).
Make a list of arrays (two-dimensional) and multiply all the elements by 100.
- Construct a single-dimensional array of integers, convert all the elements into String, and then add a suffix to them (if an element of your array is 6, make it look like 6arduino).
- Build two arrays of integers (any dimension you want). Make the rows and columns the same (if multi-dimensional). Then pide the first array with the second array, and store it into another array. Later, print them. (Hints: Suppose your arrays are
, the third array should be
.)
- Write a program that will have the following output:
