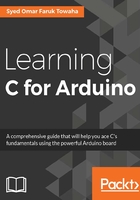
Expression in C
Before we learn about logical operations, let's look at a few expressions in C programming. Suppose you need to identify the larger of two numbers (100 and 200). You will definitely say 200 is larger than 100, right? The question is, how did you get your answer? Yes, by comparing the numbers with each other. The condition is 100<200, or 200>100. The two symbols (< and >) are known as less than and greater than. Let's look at a few more expressions:
Tip
Remember, we use double equal signs (==), not a single equal sign (=) to indicate a data type is equal to another. The preceding expressions only work when we use logical conditions in C.
Logical operations in C
Sometimes, an event may depend on something. Say, if the weather is good, I will go outside. If I can connect my Arduino to my PC properly, I will be able to code for it. If it is Sunday, I will be sleeping the whole day, and so on. All these sentences depend on certain conditions. If something happens, then something will be done.
If-statement
Look at the following figure for a clear idea:

The figure shows you two options: Yes and No. If the weather is good (Yes), then I will go outside, and if the weather is bad (No), I won't go outside. Remember, the main logic here is, if an something occurs, something else will be done or not done. In Arduino for C, we call this logical operation an if-condition.
Let's look at an example on our Arduino IDE.
Fire up your Arduino IDE and write the following code first. I will explain the code a little bit later:
String condition; void setup() { Serial.begin(9600); condition = "No"; // set condition's value if (condition == "Yes") { Serial.println("I will go outside"); } if (condition == "No") { Serial.println("I will not go outside"); } } void loop() { }
Can you guess the output from the code? If yes, then you absolutely know what an if-statement is. If not, let's dissect the code now.
On the first line, we declared a variable, condition
, which is a string
. Inside our setup()
function, we set the value of condition
to No
. In C, we already have a built-in control statement, known as if-statement. Inside our if()
function, we passed our condition, which is equal to yes
. The basic syntax for the if-condition is as follows:
if(condition){ ------statements------; }
Inside the curly brackets, we write our statements, which will be executed if the condition inside the if()
function is true; otherwise, the statement will not be executed. In our example, we passed our condition
variable's value as yes, which is not true because we declared our condition to be No
. As we stated before, if the condition is false, the statement inside the curly brackets will not be executed.
If we set the condition
value to No
, then our statement inside the curly brackets will be executed. In the example, the first if condition is false but the second is true. So, the statement inside the second if will be executed. Let's look at the output of the code:

Yes, our code has the final output I will not go outside
.
If our block has one statement, we can write the statement directly after the if()
function. This will not require any curly brackets. Our example can be written as follows:
if (condition == "Yes") Serial.println("I will go outside");
But if we have multiple statements, we must place our statements inside the curly brackets. Look at the following example:
if(X>Y){ Serial.println("Ha ha ha!"); Serial.println("I knew it"); Serial.println("X is greater than Y"); }
My suggestion is, always use the curly brackets so that you don't need to worry about when to skip brackets or not.
Let's look at another example. Let's say John and Ron are two farmers. They grow flowers. But they are lazy. They hardly take responsibility for selling the flowers. So, they made a pact, which is, if John grows more than 300 flowers, Ron is responsible for selling them. If Ron grows fewer than 200 flowers, John is responsible for selling them. If the number of flowers is the same same, then both will go out selling flowers. One day, John grew 312 flowers and Ron grew 256 flowers. Now, since we know the if condition, let's write a program to find out who will be selling flowers.
First, we need to declare two variables for the number of flowers grown by John and Ron. Let's say they are jFlower
and rFlower
. Now, assign the values to them as follows:
int jFlower = 312; int rFlower = 256;
You might remember from the previous chapter why we made our variables integers.
Now, let's look at the conditions:
- if jFlower is greater than 300, Ron will be selling the flowers
- if rFlower is less than 200, John will be selling the flowers.
- if jFlower and rFlower are equal, both John and Ron will sell the flowers.
For the three conditions, we need to define three if-conditions:
//First if(jFlower > 300){ Serial.println("Ron will sell flower today"); } //Second if(rFlower < 200){ Serial.println("John will sell flower today"); } //Third if(jFlower == rFlower){ Serial.println("Both John and Ron will sell flower today"); }
From the initialization of the two variables ( jFlower
and rFlower
), we can definitely say which condition will be executed. Let's see if your answer is right. Now, fire up your Arduino IDE and the full code given here:
int jFlower = 312; int rFlower = 256; void setup() { Serial.begin(9600); if (jFlower > 300) { Serial.println("Ron will sell flower today"); } if (rFlower < 200) { Serial.println("John will sell flower today"); } if (jFlower == rFlower) { Serial.println("Both John and Ron will sell flower today"); } } void loop() { }

Yes, your answer was right! Ron will be selling flowers today! Let's make the problem even more complex. But before doing that, let's learn about something known as nested if
.
Nested if
Have you seen a bird's nest? Birds make their nests by collecting leaves, grass, twigs, and so on. But, for most birds, have you noticed they start building the outside of the nest by putting things one over another, and later, they make the nest by surrounding it with small things from inside. Look at the following figure:
http://tse4.mm.bing.net/th?id=OIP.M9b6c52170fba2232051db1ce4b62b9a7o0
This is a bird nest made of small, natural objects. There are long leaves both inside the nest and outside the nest, right?
Our nested if is similar to the bird's nest. If an if-statement stays inside another if-statement, the whole structure is known as a nested if-statement. The basic syntax of the nested if looks as follows:
if(condition){ --------statements------- if(condition){ --------statements------- } }
Remember, there can be multiple if-statements in a nested if. First let's look at the logical operations for a nested if with two if conditions.
Suppose you want to be a member of a club that requires two conditions to be met. You must be over 18 years of age, and you must spend $200 on membership.
If you break any of the two conditions, you cannot have the membership. So, we cannot just use two if conditions. Let's look at what happens if we use a general if-statement other than nested if:
First, we need to declare two variables, as follows:
int subscription; //This variable will hold the amount that you want to spend int age; // this variable will contain your age.
Now we will assign the values. Say, my age is 12 but I want to spend $200 for the membership. See, the subscription amount fulfills the condition of the club, but the age does not. So, the club won't let me be a member of the club. Let's assign values to our variables and write two general if-statements:
subscription = 200; age = 12; if(subscription ==200){ Serial.println("You can be our member!"); } if(age>=18){ Serial.println("You can be our member!"); }
Now, run the code and look at the output:

Our output shows only one condition's statement. What happened to the other? It has not been executed due to not being the correct condition. But, we do not want that. We want both the conditions to fulfill the club's requirements so a person can get membership. To check if both the if-statements are executed at the same time, we can use nested if.
If the outer and inner if-statements both fulfill the conditions, our problem can be solved. Let's write the code using nested-if:
int subscription; int age; void setup() { subscription = 200; age = 12; Serial.begin(9600); if (subscription == 200) { if (age >= 18) { Serial.println("You can be our member!!"); } } } void loop() { }
The output looks as follows:

Our output is blank. Why? Because only one condition is fulfilled. If both were true, then we would get the correct result. Let's change the value of age to 30 and run the code again. The output of our code is as follows:

Now our code shows what we expected. Both conditions are fulfilled and we have achieved our desired output.
Can you guess the output of the following code?
int subscription; int age; void setup() { subscription = 250; age = 20; Serial.begin(9600); if (subscription == 200) { if (age >= 18) { Serial.println("1. You can be our member!!"); } } if (subscription == 200) { if (age <= 10) { Serial.println("2. You can be our member!!"); } } if (subscription == 100) { if (age >= 18) { Serial.println("3. You can be our member!!"); } } if (subscription > 100) { if (age == 18) { Serial.println("4. You can be our member!!"); } } if (subscription < 100) { if (age > 18) { Serial.println("5. You can be our member!!"); } } if (subscription > 100) { if (age > 10) { Serial.println("6. You can be our member!!"); } } } void loop() { }
The output is as follows:
