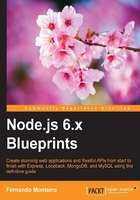
Changing the application's structure
Let's make some changes to the structure of directories in our application and prepare it to follow the Model-View-Controller design pattern.
I will list the necessary steps for this refactoring:
- Inside the
root
project folder:- Create a new folder called
server
- Create a new folder called
- Inside the
server
folder:- Create a new folder called
config
- Create a new folder called
routes
- Create a new folder called
views
.
- Create a new folder called
- Do not worry about the
config
folder at this point; we will insert its contents later. - Now we need to move the
error.js
andindex.js
files from thechapter-01/views
folder to thechapter-01/server/views
folder. - Move the
index.js
anduser.js
files from thechapter-01/routes
folder to thechapter-01/server/routes
folder. - A very simple change here, but during the development process, it will be very useful to better organize all the files of our application.
We still need to change the path to this folder in the main application file, app.js
. Open the app.js
file from the project root folder and change the following highlighted lines:
... var routes = require('./server/routes/index'); var users = require('./server/routes/users'); var app = express(); // view engine setup app.set('views', path.join(__dirname, 'server/views')); app.set('view engine', 'ejs'); ...
Before we proceed, let's change the welcome message from the routes/index.js
file to the following highlighted code:
/* GET home page. */
router.get('/', function(req, res, next) {
res.render('index', { title: 'Express from server folder' });
});
To run the project and see the application in your browser, follow these steps:
- Type the following command in your terminal/shell:
DEBUG=chapter-01:* npm start
- Open your browser at
http://localhost:3000
. - The output in your browser will be as follows:
Application home screen
Now we can delete the folders and files from:
chapter-01/routes
:-
index.js
-
user.js
-
chapter-01/views
:error.js
-
index.js
Changing the default behavior to start the application
As mentioned earlier, we will change the default initialization process of our application. To do this task, we will edit the app.js
file and add a few lines of code:
- Open
app.js
and add the following code after theapp.use('/users', users);
function:// catch 404 and forward to error handler app.use(function(req, res, next) { var err = new Error('Not Found'); err.status = 404; next(err); });
It's a simple
middleware
to intercept 404 errors. - Now add the following code after the
module.exports = app;
function:app.set('port', process.env.PORT || 3000); var server = app.listen(app.get('port'), function() { console.log('Express server listening on port ' + serer.address().port); });
- Open the
package.js
file at the root project folder and change the following code:... "scripts": { "start": "node app.js" }, ...
Note
The debug command is still available if necessary:
DEBUG=chapter-01:* npm start
. - The
package.json
file is a file of extreme importance in Node.js applications. It is possible to store all kinds of information for the project, such as dependencies, project description, authors, version, and many more. - Furthermore, it is possible to set up scripts to minify, concatenate, test, build and deploy an application easily. We'll see more on how to create scripts in Chapter 9, Building a Frontend Process with Node.js and NPM.
- Let's test the result; open your terminal/shell and type the following command:
npm start
- We will see the same output on the console:
> node app.js Express server listening on port 3000!