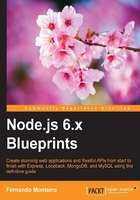
上QQ阅读APP看书,第一时间看更新
Adding templates for login, sign-up, and profile
Now we have a solid basis to move forward with the project. At this time, we will add some template files for login, sign-up, and profile screens.
The expected result for these pages will be as shown in the following screenshot:

Login screen

Sign-up screen

Profile screen
- Now let's create the login template. Create a new file called
login.ejs
in theviews
folder and place the following code:<!DOCTYPE html> <html> <head> <title><%= title %></title> <% include ../partials/stylesheet %> </head> <body> <% include ../partials/header %> <div class="container"> <% if (message.length > 0) { %> <div class="alert alert-warning alert-dismissible fade in" role="alert"> <button type="button" class="close" data-dismiss= "alert" aria-label="Close"> <span aria-hidden="true">×</span> </button> <strong>Ohps!</strong> <%= message %>. </div> <% } %> <form class="form-signin" action="/login" method="post"> <h2 class="form-signin-heading">Welcome sign in</h2> <label for="inputEmail" class="sr-only">Email address</label> <input type="email" id="email" name="email" class="form- control" placeholder="Email address" required=""> <label for="inputPassword" class="sr-only">Password</label> <input type="password" id="password" name="password" class="form-control" placeholder="Password" required=""> <button class="btn btn-lg btn-primary btn-block" type="submit">Sign in</button> <br> <p>Don't have an account? <a href="/signup">Signup</a> ,it's free.</p> </form> </div> <% include ../partials/footer %> <% include ../partials/javascript %> </body> </html>
- Add the login route to
routes/index.js
after the index route:/* GET login page. */ router.get('/login', function(req, res, next) { res.render('login', { title: 'Login Page', message: req.flash('loginMessage') }); });
Note
In the template, we are making use of the
connect-flash
middleware to display error messages. Later, we will show how to install this component; don't worry right now. - Let's add the
signup
template to theviews/pages
folder. - Create a new file in
views/pages
and save assignup.ejs
; then add the following code:<!DOCTYPE html> <html> <head> <title><%= title %></title> <% include ../partials/stylesheet %> </head> <body> <% include ../partials/header %> <div class="container"> <% if (message.length > 0) { %> <div class="alert alert-warning" role="alert"> <strong>Warning!</strong> <%= message %>. </div> <% } %> <form class="form-signin" action="/signup" method="post"> <h2 class="form-signin-heading">Please signup</h2> <label for="inputName" class="sr-only">Name address</label> <input type="text" id="name" name="name" class="form-control" placeholder="Name" required=""> <label for="inputEmail" class="sr-only">Email address</label> <input type="email" id="email" name="email" class= "form-control" placeholder="Email address" required=""> <label for="inputPassword" class="sr-only">Password</label> <input type="password" id="password" name="password" class="form-control" placeholder="Password" required=""> <button class="btn btn-lg btn-primary btn-block" type="submit">Sign in</button> <br> <p>Don't have an account? <a href="/signup">Signup</a> ,it's free.</p> </form> </div> <% include ../partials/footer %> <% include ../partials/javascript %> </body> </html>
- Now we need to add the route for the sign-up view. Open
routes/index.js
and add the following code right afterlogin route
:/* GET Signup */ router.get('/signup', function(req, res) { res.render('signup', { title: 'Signup Page', message:req.flash('signupMessage') }); });
- Next, we will add the template to the
profile
page and the route to this page. Create a file calledprofile.ejs
inside theview/pages
folder and add this code:<!DOCTYPE html> <html> <head> <title><%= title %></title> <% include ../partials/stylesheet %> </head> <body> <% include ../partials/header %> <div class="container"> <h1><%= title %></h1> <div class="datails"> <div class="card text-xs-center"> <br> <img class="card-img-top" src="<%= avatar %>" alt="Card image cap"> <div class="card-block"> <h4 class="card-title">User Details</h4> <p class="card-text"> <strong>Name</strong>: <%= user.local.name %><br> <strong>Email</strong>: <%= user.local.email %> </p> <a href="/logout" class="btn btn-default">Logout</a> </div> </div> </div> </div> <% include ../partials/footer %> <% include ../partials/javascript %> </body> </html>
- Now we need to add the route for the profile view; open
routes/index.js
and add the following code right after thesignup
route:/* GET Profile page. */ router.get('/profile', function(req, res, next) { res.render('profile', { title: 'Profile Page', user : req.user, avatar: gravatar.url(req.user.email , {s: '100', r: 'x', d: 'retro'}, true) }); });
Tip
We are using another middleware called
gravatar
; later, we will show how to install it.