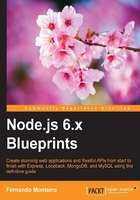
上QQ阅读APP看书,第一时间看更新
Creating the application controllers
The next step is to create the controls for the models User and Band:
- Within the
controllers
folder, create a new file calledUser.js
and add the following code:var models = require('../models/index'); var User = require('../models/user'); // Create Users exports.create = function(req, res) { // create a new instance of the Users model with request body models.User.create({ name: req.body.name, email: req.body.email }).then(function(user) { res.json(user); }); }; // List Users exports.list = function(req, res) { // List all users models.User.findAll({}).then(function(users) { res.json(users); }); };
Tip
Note that the first line of the file imports the
index
model; this file is the basis for creation of all the controls, it is thesequelize
that is used to map the other models. - Do the same for the Band controller within the
controllers
folder; create a file calledBand.js
and add the following code:var models = require('../models/index'); var Band = require('../models/band'); // Create Band exports.create = function(req, res) { // create a new instance of the Bands model with request body models.Band.create(req.body).then(function(band) { //res.json(band); res.redirect('/bands'); }); }; // List Bands exports.list = function(req, res) { // List all bands and sort by Date models.Band.findAll({ // Order: lastest created order: 'createdAt DESC' }).then(function(bands) { //res.json(bands); // Render result res.render('list', { title: 'List bands', bands: bands }); }); }; // Get by band id exports.byId = function(req, res) { models.Band.find({ where: { id: req.params.id } }).then(function(band) { res.json(band); }); } // Update by id exports.update = function (req, res) { models.Band.find({ where: { id: req.params.id } }).then(function(band) { if(band){ band.updateAttributes({ name: req.body.name, description: req.body.description, album: req.body.album, year: req.body.year, UserId: req.body.user_id }).then(function(band) { res.send(band); }); } }); } // Delete by id exports.delete = function (req, res) { models.Band.destroy({ where: { id: req.params.id } }).then(function(band) { res.json(band); }); }
- Now let's refactor the
index.js
controller and add the following code:// List Sample Bands exports.show = function(req, res) { // List all comments and sort by Date var topBands = [ { name: 'Motorhead', description: 'Rock and Roll Band', album: 'http://s2.vagalume.com/motorhead/discografia /orgasmatron-W320.jpg', year:'1986', }, { name: 'Judas Priest', description: 'Heavy Metal band', album: 'http://s2.vagalume.com/judas-priest/discografia /screaming-for-vengeance-W320.jpg', year:'1982', }, { name: 'Ozzy Osbourne', description: 'Heavy Metal Band', album: 'http://s2.vagalume.com/ozzy-osbourne/discografia /diary-of-a-madman-W320.jpg', year:'1981', } ]; res.render('index', { title: 'The best albums of the eighties', callToAction: 'Please be welcome, click the button below and register your favorite album.', bands: topBands }); };
Note that, using the previous code, we just created a simple list to show some albums on the home screen.