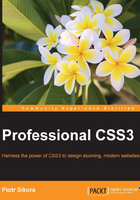
Traditional box model
An understanding of the box model is the foundation of CSS theories. You have to know the impact of width, height, margin, and borders on the size of the box and how you can manage it to match the elements on a website. The main questions for coders and frontend developers in interviews are based on box model theories. Let's begin this important chapter, which will be the foundation for every upcoming subject.
Padding/margin/border/width/height
The ingredients of the final width and height of the box are as follows:
- Width
- Height
- Margins
- Paddings
- Borders
For better understanding of the box model, the following is an image from Google Chrome inspector:

For more clarity and better understanding of the box model, let's analyze the following image:

In the preceding image, you can see that in the box model, we have the following four edges:
- Content edge
- Padding edge
- Border edge
- Margin edge
The width and height of the box are based on the following:
- Width/height of content
- Padding
- Border
- Margin
The width and height of content in the box with default box-sizing are controlled by the following properties:
- Min-width
- Max-width
- Width
- Min-height
- Max-height
- Height
An important thing about the box model is how background properties will behave. The background will be included in the content section and in the padding section (to the padding edge).
Let's get a code and try to point to all elements of the box model.
HTML code:
<div class="element"> Lorem ipsum dolor sit amet consecteur </div>
CSS code:
.element { background: pink; padding: 10px; margin: 20px; width: 100px; height: 100px; border: solid 10px black; }
In the browser, we will see the following:

The view from the inspector of Google Chrome is as follows:

Let's check how the areas of the box model are placed in the following specific example:

The basic task for the interviewed frontend developer is:
The box/element is described with the styles:
.box { width: 100px; height: 200px; border: 10px solid #000; margin: 20px; padding: 30px; }
Please count the final width
and height
(the real space which is needed for this element) of this element.
So, as you can see, the problem is to count the width and height of the box.
The ingredients of width are as follows:
- Width
- Border left
- Border right
- Padding left
- Padding right
Additionally for the width of space taken by the box:
- Margin left
- Margin right
The ingredients of height are as follows:
- Height
- Border top
- Border bottom
- Padding top
- Padding bottom
Additionally for height of space taken by the box:
- Margin top
- Margin bottom
Therefore, when you sum the elements, you will have the following equations:
Width:
Box width = width + borderLeft + borderRight + paddingLeft + paddingRight Box width = 100px + 10px + 10px + 30px + 30px = 180px
Space width:
width = width + borderLeft + borderRight + paddingLeft + paddingRight + marginLeft + marginRight width = 100px + 10px + 10px + 30px + 30px + 20px + 20 px = 220px
Height:
Box height = height + borderTop + borderBottom + paddingTop + paddingBottom Box height = 200px + 10px + 10px + 30px + 30px = 280px
Space height:
Space height = height + borderTop + borderBottom + paddingTop + paddingBottom + marginTop + marginBottom Space height = 200px + 10px + 10px + 30px + 30px + 20px + 20px = 320px
You can check it in the real browser as shown in the following:

Omitting problems with the traditional box model (box-sizing)
Basic theory of the box model is pretty hard to learn. You need to remember all the elements of width/height, even if you set the width and height. The hardest thing for beginners to understand is padding, which shouldn't be counted as a component of width and height. It should be inside the box and it should impact on this value. To change these behaviors with CSS3, supported since Internet Explorer 8, comes box-sizing.
You can set the value as follows:
box-sizing: border-box
What does it give to you? Finally, the counting of box width and height will be easier because box padding and the border are inside the box. So if we are taking our previous class:
.box { width: 100px; height: 200px; border: 10px solid #000; margin: 20px; padding: 30px; }
We can count the width and height easily:
Width = 100px Height = 200px
Additionally, the space taken by the box:
- Space width = 140px (because the 20px margin is on both sides left and right)
- Space height = 240px (because the 20px margin is on both sides top and bottom)
The following is a sample from Google Chrome:

Therefore, if you do not want to repeat all the problems of the traditional box model, you should use it globally for all elements. Of course, it's not recommended for old projects, for example, a new client who needs some small changes in the old project. If you add the following code:
* { width: 100px; }
You can cause more harm than good because of the inheritance of this property for all elements, which are now based on the traditional box model. But for all new projects, you should use it.