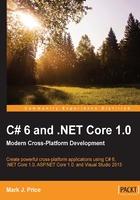
Iteration statements
Iteration statements repeat a block either while a condition is true
or for each item in a sequence. The choice of which statement to use is based on a combination of ease of understanding to solve the logic problem and personal preference.
Add a new Console Application project named Ch03_IterationStatements.
Set the solution's startup project to be the current selection.
The while statement
The while
statement evaluates a Boolean expression and continues to loop while it is true
.
Type the following code inside the Main
method (remember to statically import the System.Console
type!):
int x = 0; while (x < 10) { WriteLine(x); x++; }
Press Ctrl + F5 and view the output in the console:
0 1 2 3 4 5 6 7 8 9
The do-while statement
The do-while
statement is like while
except the Boolean expression is checked at the bottom of the block instead of the top, which means that it always executes at least once.
If you want to try the code for the do-while
statement, then select all the previous statements and press Ctrl + K, C to comment them out and then enter the following code and run it:
int x = 0; do { WriteLine(x); x++; } while (x < 10);
When you then press Ctrl + F5, you will see that the results are the same as those we got earlier.
The for statement
The for
statement is like while
except that it is more succinct. It combines an initializer statement that executes once at the start of the loop, a Boolean expression to check whether the loop should continue, and an incrementer that executes at the bottom of the loop.
The for
statement is commonly used with an integer counter, although it doesn't have to be as shown in the following code:
for (int y = 0; y < 10; y++) { WriteLine(y); }
The foreach statement
The foreach
statement is a bit different from the other three. It is used to perform a block of statements on each item in a sequence (for example, an array or collection). Each item is read-only and if the sequence is modified during iteration, for example, by adding or removing an item, then an exception will be thrown.
Type the following code inside the Main
method, which creates an array of string
variables and then uses a foreach
statement to enumerate and output the length of each of them:
string[] names = { "Adam", "Barry", "Charlie" }; foreach (string name in names) { WriteLine($"{name} has {name.Length} characters."); }
Press Ctrl + F5 and view the output in the console:
Adam has 4 characters. Barry has 5 characters. Charlie has 7 characters.
How does the foreach statement actually work?
Technically, the foreach
statement will work on any type that implements an interface called IEnumerable
, but you don't need to worry about what an interface is for now. You will learn about interfaces in Chapter 7, Implementing Interfaces and Inheriting Classes.
If you use a tool like ildasm
, then you will see that the compiler turns the foreach
statement in the preceding code into something like this:
IEnumerator e = names.GetEnumerator(); while(e.MoveNext()) { string name = (string)e.Current; // Current is read-only! WriteLine($"{name} has {name.Length} characters."); }
Tip
Due to the use of an iterator, the variable declared in a foreach
statement cannot be used to modify the value of the current item.