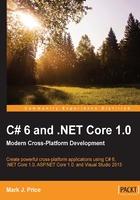
Practice and explore
Test your knowledge and understanding by answering some questions, get some hands-on practice, and explore with deeper research into this chapter's topics.
Exercise 3.1 – test your knowledge
Answer the following questions:
- What happens when you pide an
int
variable by0
? - What happens when you pide a
double
variable by0
? - What happens when you overflow an
int
variable, that is, set it to a value beyond its range? - What is the difference between
x = y++;
andx = ++y;
? - What is the difference between
break
,continue
, andreturn
when used inside aloop
statement? - What are the three parts of a
for
statement and which of them are required? - What is the difference between the
=
and==
operators?
Exercise 3.2 – explore loops and overflow
What will happen if this code executes?
int max = 500; for (byte i = 0; i < max; i++) { WriteLine(i); }
Add a new Console Application named Ch03_Exercise02 and enter the preceding code. Run the application by pressing Ctrl + F5. What happens?
What code could you add (don't change any of the preceding code) to warn us about the problem?
Exercise 3.3 – practice loops and operators
FizzBuzz is a group word game for children to teach them about pision. Players take turns to count incrementally, replacing any number pisible by three with the word "fizz", any number pisible by five with the word "buzz", and any number pisible by both with "fizzbuzz".
Some interviewers give applicants simple FizzBuzz-style problems to solve during interviews. Most good programmers should be able to write out on paper or whiteboard a program to output a simulated FizzBuzz game in under a couple of minutes.
Want to know something worrisome? Many computer science graduates can't. You can even find senior programmers who take more than 10-15 minutes to write a solution.

This quote is taken from http://blog.codinghorror.com/why-cant-programmers-program/.
Refer to the following link for more information:
http://imranontech.com/2007/01/24/using-fizzbuzz-to-find-developers-who-grok-coding/
Create a Console Application named Ch03_Exercise03 that outputs a simulated FizzBuzz game counting up to 100
. The output should look something like this:
1, 2, Fizz, 4, Buzz, Fizz, 7, 8, Fizz, Buzz, 11, Fizz, 13, 14, FizzBuzz, 16, 17, Fizz, 19, Buzz, Fizz, 22, 23, Fizz, Buzz, 26, Fizz, 28, 29, FizzBuzz, 31, 32, Fizz, 34, Buzz, Fizz, 37, 38, Fizz, Buzz, 41, Fizz, 43, 44, FizzBuzz, 46, 47, Fizz, 49, Buzz, Fizz, 52, 53, Fizz, Buzz, 56, Fizz, 58, 59, FizzBuzz, 61, 62, Fizz, 64, Buzz, Fizz, 67, 68, Fizz, Buzz, 71, Fizz, 73, 74, FizzBuzz, 76, 77, Fizz, 79, Buzz, Fizz, 82, 83, Fizz, Buzz, 86, Fizz, 88, 89, FizzBuzz, 91, 92, Fizz, 94, Buzz, Fizz, 97, 98, Fizz, Buzz
Exercise 3.4 – practice exception handling
Create a Console Application named Ch03_Exercise04 that asks the user for two numbers in the range 0
-255
and then pides the first number by the second:
Enter a number between 1 and 255: 100 Enter another number between 1 and 255: 8 100 pided by 8 is 12
Write exception handlers to catch any thrown errors:
Enter a number between 1 and 255: apples Enter another number between 1 and 255: bananas FormatException: Input string was not in a correct format.
Exercise 3.5 – explore topics
Use the following links to read in more detail about the topics covered in this chapter:
- Selection Statements (C# Reference): https://msdn.microsoft.com/en-us/library/676s4xab.aspx
- Iteration Statements (C# Reference): https://msdn.microsoft.com/en-us/library/32dbftby.aspx
- Jump Statements (C# Reference): https://msdn.microsoft.com/en-us/library/d96yfwee.aspx
- Casting and Type Conversions (C# Programming Guide): https://msdn.microsoft.com/en-us/library/ms173105.aspx
- Exception Handling Statements (C# Reference): https://msdn.microsoft.com/en-us/library/s7fekhdy.aspx
- Checked and Unchecked (C# Reference): https://msdn.microsoft.com/en-us/library/khy08726.aspx
- Namespace Keywords (C# Reference): https://msdn.microsoft.com/en-us/library/cxtk6h5e.aspx
- StackOverflow: http://stackoverflow.com/
- Google Advanced Search: http://www.google.com/advanced_search
- Design Patterns: https://msdn.microsoft.com/en-us/library/ff649977.aspx
- patterns & practices: https://msdn.microsoft.com/en-us/library/ff921345.aspx