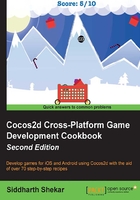
Animating sprites
In this section, we will discuss how to animate a sprite. We will change our custom sprite class to make the character animate. This can be done by providing Cocos2d with a number of images and asking it to cycle through these images to create the animation.
Getting ready
To animate the sprites, we will add four frames of animation that we will apply to the hero
sprite class and cycle through the images using the repeatForever
action. In the next section, we will cover the actions in detail.
How to do it…
In the Resources
folder for this chapter, there will be normal, ipad
, and ipadhd
versions of the frames for hero
. We will import all the files into the project.

In the Hero.m
file, we will change the initWithFilename
function, as follows:
-(id)initWithFilename:(NSString *)filename { if (self = [super initWithImageNamed:filename]) { NSMutableArray *animFramesArray = [NSMutableArray array]; for (inti=1; i<=4; i++){ [animFramesArrayaddObject: [CCSpriteFrameframeWithImageNamed: [NSStringstringWithFormat:@"hero%d.png",i ]]]; } CCAnimation* animation = [CCAnimation animationWithSpriteFrames:animFramesArraydelay:0.3]; CCActionInterval *animate= [CCActionAnimateactionWithAnimation:animation]; CCAction* repeateAnimation = [CCActionRepeatForeveractionWithAction:animate]; [selfrunAction:repeateAnimation]; } return self; } @end
First, we will create a new variable called animFramesArray
of the NSMutableArray
type.
We will then create a for
loop starting from index 1
and going to 4
, as there are four images. We will save the frames in the array by passing in the names of the four images that we would like to loop through.
Next, we create a variable called animation
of the CCAnimation
type and pass it in the four frames; also add the delay with which the animation should be played with.
We will then create a variable called animate
of the CCActionInterval
type to create a loop through the animation. Then, in the next step, we will create CCAction
and call the repeat forever
action, which will loop through the animation forever.
Finally, we will run the action on the current class.
How it works…
Now, you should be able to see the character blinking. The speed with which the animation is played is controlled by the delay in the CCAnimation
class.

We will see that without making any changes to the instance in MainScene
, the custom sprite animates the character.