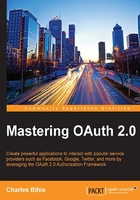
Step 1 – Register your client application
Before you start making requests to an OAuth 2.0 service provider, the service provider must first know who you are. This is what the registration process is for. The registration process does a lot of things, but most importantly, it establishes a trust relationship between your application and the service provider so that, once established, your application can communicate effectively with the service provider. This is a one-time process and must be done at the beginning of your integration. Once you've registered, you won't have to repeat this step for the lifetime of your application (although you may have to revisit the configurations you set up during this step as your needs and settings may change as your application evolves). Here is a brief list of what is accomplished during the registration process and why:
- You identify your client application: This can be as simple as a name, and is used to distinguish your application from all of the others.
Note
Why is this important?
Without identifying your application, the service provider wouldn't know who is making requests, and, therefore, wouldn't know if your application is allowed or not.
If a particular client starts acting inappropriately, the service provider is able to revoke access to that client without impacting the service for anyone else.
Let's look at an example. Consider signing up for a gym. Before being able to use the facilities, the gym needs to know who you are so that they can determine if you have access or not. Furthermore, if you start misbehaving, the gym can revoke access for just your key card without impacting any other gym members.
- You give necessary details about your client application: There are certain properties of your client application that the service provider would need to know in order to communicate effectively. For instance, in Chapter 2, A Bird's Eye View of OAuth 2.0, we stated that the capabilities of your application determine the workflow that will be used (authorization code grant versus implicit grant).
Note
Why is this important?
If the service provider doesn't know how your application is set up, it won't be able to communicate with it.
Let's now look at an example regarding communication. The gym would need to know your contact details and contact preferences. Without this, the gym wouldn't know how to send you information. Or worse yet, would send that information to the wrong people.
Different service providers, different registration process, same OAuth 2.0 protocol
When registering with different OAuth 2.0 service providers, you will notice that each provider has a unique registration process, and often each requires different pieces of information about your client. However, they are all powered by the same OAuth 2.0 protocol. So, while there are definitely service-provider-specific properties that differ with each provider, there is a base set of information that you should be able to walk away with. This messy process can be visualized with the following diagram:

As you can see, the intake for each different service provider varies wildly, depending on the company and how they choose to manage their applications. However, in the end, you should expect a strict set of properties and endpoints that will be necessary for you to start integrating. The set of properties that you should have after registering your application includes the following:
- Client ID: This is your client application's unique identifier. Depending on the provider, sometimes this will be generated for you, and other times, you can specify it yourself.
Don't get this confused with your client name, which is just the human-readable name for your application and does not have to be unique. Your client ID must be unique across the entire application space of the service provider.
- Client secret: This is your secret key for your application and is used to identify itself when making requests. This will always be issued to you by the service provider.
Tip
Only for trusted clients
If you are using the implicit grant, you may not get a client secret since untrusted clients aren't able to securely store this value.
- Redirection endpoint: This is an endpoint that the service provider will use to send you responses (tokens or errors, usually). Most of the time, this will be provided by you. But in certain cases, such as with installed desktop or native mobile applications, this can be determined by the provider.
- Authorization endpoint: This is an endpoint that your client application will use to initiate the authorization flow. This will be determined by the service provider.
- Token endpoint: This is an endpoint that your client application will use to initiate token flows. This will also be determined by the service provider.
Even though the registration process is different from service provider to service provider, at the end of the process, you should walk away with these five properties.
Here is an example of what those properties may look like for our GoodApp application:
- Client ID:
goodapp-541106
- Client secret:
38D83HHFF873RASDPPEKJ1KHJZL
- Redirection endpoint:
https://www.goodapp.com/callback
- Authorization endpoint:
https://api.facebook.com/auth
- Token endpoint:
https://api.facebook.com/token
With these five pieces of information, we have all that we need to proceed with our integration.
Your client credentials
You will hear references to the term client credentials as we proceed with our discussion of OAuth 2.0. Your client credentials are essentially your client ID and client secret. Combined, these are used to identify your application to the service provider. You can think of this as the equivalent of a username and password, but for your application. This is to ensure that the service provider can know who they are delegating authorization to, so that they don't give your friend list to the wrong application.
If your credentials ever get leaked, it is important to change them immediately. Otherwise, this would allow another application (or person) to masquerade as your application, which can potentially have some very devastating results.
Tip
Best practice
Just as with your own personal credentials for various accounts and websites, you should rotate your client credentials as well. Set an interval, say, every 6 months, or every major release (depending on the security needs of your application, this may be longer or shorter) where you will request a new client secret and invalidate your old one. This will minimize the impact in the case that your client secret gets leaked.