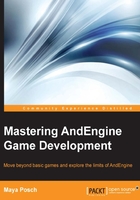
Application basics
The base for building an AndEngine application is the BaseGameActivity
class, which we'll use instead of the standard Activity
class. The former provides the base functionality, which we'll need in our application. There's also the SimpleBaseGameActivity
class, which exists for compatibility with GLES1. Unlike GLES2's BaseGameActivity
, it does not use callbacks—methods called by AndEngine that we define ourselves—and uses a more basic setup and configuration model. As we're not using GLES1, we are not interested in using this class.
The BaseGameActivity
class has four functions that we override for our own functionality:
onCreateEngineOptions()
onCreateResources
(OnCreateResourcesCallback
,pOnCreateResourcesCallback
)onCreateScene
(OnCreateSceneCallback
,pOnCreateSceneCallback
)onPopulateScene
(Scene
pScene
,OnPopulateSceneCallback
,pOnPopulateSceneCallback
)
The advantage of the GLES2 base class is that through the calling of the callback in each overridden function, you can explicitly proceed to the next function in the list instead of implicitly having all of them called. Other than this, very little changes. In the onCreateEngineOptions()
function, we still create a Camera object and assign it together with the parameters for the screen orientation and ratio to an EngineOptions
object, which is returned. No callback is called here yet.
The remaining three functions respectively load the resources for the application, create the scene, and populate the said scene. A basic application skeleton thus looks like this:
public class MainActivity extends BaseGameActivity { private Scene mScene; private static final int mCameraHeight = 480; private static final int mCameraWidth = 800; @Override public EngineOptions onCreateEngineOptions() { Camera mCamera = new Camera(0, 0, mCameraWidth, mCameraHeight); final EngineOptions engineOptions = new EngineOptions(true, ScreenOrientation.LANDSCAPE_FIXED, new RatioResolutionPolicy( mCameraWidth, mCameraHeight), mCamera); return engineOptions; } @Override public void onCreateResources( OnCreateResourcesCallback pOnCreateResourcesCallback) throws IOException { // Load any resources here pOnCreateResourcesCallback.onCreateResourcesFinished(); } @Override public void onCreateScene(OnCreateSceneCallback pOnCreateSceneCallback) throws IOException { mScene = new Scene(); pOnCreateSceneCallback.onCreateSceneFinished(mScene); } @Override public void onPopulateScene(Scene pScene, OnPopulateSceneCallback pOnPopulateSceneCallback) throws IOException { // Populate the Scene here pOnPopulateSceneCallback.onPopulateSceneFinished(); } }