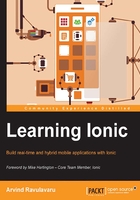
The Ionic project structure
So far, we have scaffolded a blank Ionic app and launched it in a browser. Now, we will walk through the scaffolded project structure.
To quickly remind you, we know that the Ionic sits inside the Cordova application. So before we go to the Ionic code, we will talk about the Cordova wrapper.
If you open the chapter2 example1
folder in your text editor, you should see the following folder structure at the root of the project:
. ├── bower.json ├── config.xml ├── gulpfile.js ├── hooks ├── ionic.project ├── package.json ├── plugins ├── scss └── www
Here is a quick explanation of each of the items:
bower.json
: This consists of the dependencies loaded via Bower. In future, we will install other Bower packages to be used along with our app. All the information regarding the same will be available here.config.xml
: This file consists of all the meta information needed by Cordova while converting your Ionic app to a platform-specific installer. If you open config.xml, you will see a bunch of XML tags that describes your project. We will take a look at this file in detail again.gulpfile.js
: This file consists of the build tasks that we would be using while developing the Ionic app.ionic.project
: This file consists of the information regarding the Ionic app.hooks
: This folder consists of scripts that get executed when a particular Cordova task is performed. A Cordova task can be any of the following:after_platform_add
(after a new platform is added),after_plugin_add
(after a new plugin is added),before_emulate
(before emulation begins),after_run
(before the app run), and so on. Each task is placed inside a folder named after the Cordova task. If you open thehooks
folder, you will see anafter_prepare
folder and aREADME.md
file. Inside theafter_prepare
folder, you will find a script file named010_add_platform_class.js
. This will get executed after the prepare task of Cordova is executed. All that this task does is add a class to the<body>
tag, which is the same name as the platform on which the app is running. This would help us in styling the app better, based on the platform. You can find a list of tasks that you can hook into from theREADME.md
file present inside thehooks
folder.plugins
: This folder consists of all the plugins added to the project. We will be adding a few more plugins later on, and you can see them reflected here.scss
: This folder consists of the basescss
file which we will be overwriting to customize the styles of the Ionic components. More on this in Chapter 4, Ionic and SCSS.www
: This folder consists of the Ionic code. Anything you write inside this folder is intended to land inside the web view. This is where we will be spending most of our time.
The config.xml file
The config.xml
file is a platform agnostic configuration file. As mentioned earlier, this file consists of all the information needed by Cordova to convert the code in the www
folder to the platform-specific installer.
Setting up of the config.xml
file is based on the W3C's Packaged Web Apps (Widgets) specification (http://www.w3.org/TR/widgets/) and extended to specify core Cordova API features, plugins, and platform-specific settings. There are two types of configurations that you can add to this file. One is global, that is, common to all devices, and the other is specific to the platform.
If you open config.xml
in your text editor, the first tag you encounter is the XML root tag. Next, you can see the widget tag:
<widget id="app.example.one" version="0.0.1" xmlns="http://www.w3.org/ns/widgets" xmlns:cdv="http://cordova.apache.org/ns/1.0">
The id
specified above is the reverse domain name of your app, which we provided while scaffolding. Other specifications are defined inside the widget tag as its children. The children tags include the app name (that gets displayed below the app icon when installed on the device), app description, and author details.
It also consists of the configuration that needs to be adhered to while converting code in the www
folder to a native installer.
The content tag defines the starting page of the application. The access tag defines the URLs that are allowed to load in the app. By default, it loads all the URLs. The preference tag sets the various options as name value pairs. For instance, DisallowOverscroll
describes if there should be any visual feedback when the user scrolls past the beginning or end of the document.
You can read more about platform-specific configurations at the following links:
- Android: http://docs.phonegap.com/en/4.0.0/guide_platforms_android_config.md.html#Android%20Configuration
- iOS: http://docs.phonegap.com/en/4.0.0/guide_platforms_ios_config.md.html#iOS%20Configuration
Note
The importance for the platform-specific configurations and global configuration is same. You can read more about global configuration at http://docs.phonegap.com/en/4.0.0/config_ref_index.md.html#The%20config.xml%20File
The www folder
As mentioned earlier, this folder consists of our Ionic app, the HTML, CSS, and JS codes. If you open the www
folder, you will find the following file structure:
. ├── css │ └── style.css ├── img │ └── ionic.png ├── index.html ├── js │ └── app.js └── lib └── ionic ├── css ├── fonts ├── js ├── scss └── version.json
Let's look at each of these in detail:
index.html
: This is the application startup file. If you remember, inconfig.xml
, we pointed thesrc
on the content tag to this file. Since we are using AngularJS as our JavaScript framework, this file would ideally act as the base/first page for the Single Page Application (SPA). If you openindex.html
, you can see that the body tag has theng-app
attribute which points to the starter module defined inside thejs
/app.js
file.css
: This folder consists of styles that are specific to our app.img
: This folder consists of images that are specific to our app.js
: This folder consists of the JavaScript code specific to our app. This is where we write the AngularJS code. If you open theapp.js
file present inside this folder, you can see that an AngularJS module is set up, passing in Ionic as a dependency.lib
: This folder is where all the packages would be placed when we run bower install. When we scaffold the app, this folder comes along with it, loaded with Ionic files. If you want to download the assets again along with its dependencies, you can use thecd
command to go to theexample1
folder from your terminal/prompt and run the following command:bower install
You will see that four more folders get downloaded. These are a part of the dependencies listed in the
ionic-bower
package, present inbower.json
file, located at the root of the project.Ideally, we would not be using these libraries explicitly in our app. Rather, we would use the Ionic bundle which is built on top of these libraries.
This completes our tour of the blank template. Before we scaffold the next template, let us take a quick peek at the www/js/app.js
file.
As you can see, we are creating a new AngularJS module with the name starter
, and then we are injecting ionic
as a dependency.
The $ionicPlatform
service is injected as a dependency to the run
method. This service is used to detect the current platform, as well as handle the hardware buttons on (Android) devices. In the current context, we are using the $ionicPlatform.ready
method to be notified when the device is ready for us to perform operations on it.
It is a good practice, or rather a necessity in some cases, to include all your code inside the $ionicPlatform.ready
method. This way, your code executes only when the entire app is initialized.
So far you must have worked on AngularJS code related to the Web. But when you are dealing with Ionic, you would be working with scripts related to device features as well, inside the AngularJS code. Ionic provides us services to make these things happen in a more organized fashion. Hence, we went through the concept of custom services in Chapter 1, Ionic – Powered by AngularJS, and we will be going through Ionic Services in depth in Chapter 5, Ionic Directives and Services.