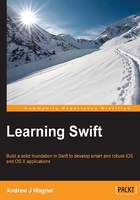
Core Swift types
Every programming language needs the ability to name a piece of information to be referenced later. This is the fundamental way that a collection of code remains readable after it is written. Swift provides a number of core types that help you represent your information in a very comprehensible way.
Constants and variables
Swift provides two types of information: a constant and a variable:
// Constant let pi = 3.14 // Variable var name = "Sarah"
All constants are defined using the let
keyword followed by a name, and all variables are defined using the var
keyword. Both the constants and variables in Swift must contain a value before they are used. This means that when you define a new constant or variable, you will most likely give it an initial value. You do so using the assignment operator (=
) followed by a value.
The only difference between the two is that a constant can never be changed, while a variable can be. In the previous example, the code defines a constant called pi
that stores the information 3.14
and a variable called name
that stores the information "Sarah"
. It makes sense to make pi
a constant because pi
will always be 3.14
. However, we want to change the value of name
in the future to something else, so we defined it as a variable.
One of the hardest parts to manage in a program is the state of all the variables. As a programmer, it can often be impossible to reason out all the different possible values a variable might have, even in relatively small programs. Especially because variables can often be changed by distant, seemingly unrelated code, so that more state will likely cause more bugs that are harder to track down. It is always best to use constants until you run into a practical scenario where you need to modify the value of the information.
Containers
It is often helpful to give a name to the more complex information we might encounter. We often deal with a related information or a series of information, such as lists. Swift provides three main collection types called tuples, arrays, and dictionaries.
Tuples
A tuple is a fixed size collection of two or more pieces of information. For example, a card in a deck of playing cards has three properties: color, suit, and value. We could use three separate variables to fully describe a card, but it would be better to express it in one:
var card = (color: "Red", suit: "Hearts", value: 7)
Each piece of information consists of a name and a value separated by a colon (:
) and each is separated by a comma (,
). Finally, the whole thing is surrounded by parentheses (()
).
Each part of a tuple can be accessed separately by name using a period (.
), which is otherwise referred to as a dot:
card.color // "Red" card.suit // "Hearts" card.value // 7
You are also able to create a tuple without names for each of its part. In that case, you can access them based on where they are in the list, zero being the first element:
var diceRoll = (4, 6) diceRoll.0 // 4 diceRoll.1 // 6
Another way to access specific values in a tuple is to capture each in a separate variable:
let (first, second) = diceRoll first // 4 second // 6
If you want to change a value within a tuple, you must reassign every value:
diceRoll = (4, 5)
Arrays
An array is essentially a variable length list of information. For example, we could create a list of people we want to invite to a party:
var invitees = ["Sarah", "Jamison", "Marcos", "Roana"]
An array always starts and ends with a square bracket and each element is separated by a comma. You can even declare an empty array with just an open and closed bracket: []
.
You can then add values to an array by adding another array to it:
invitees += ["Kai", "Naya"]
Note that +=
is the short-hand for writing:
invitees = invitees + ["Kai", "Naya"]
You can access values within an array based on their position, usually referred to as their index, in the array:
invitees[2] // Marcos
The index is specified using square brackets ([]
), which are placed immediately after the name of the array. Similar to tuples, indexes start at 0
and go up from there. So, in the preceding example, the index 2
returned the third element in the array, "Marcos"
. There is additional information you can retrieve about an array, such as the number of elements, which you will see as we move forward.
Dictionaries
A dictionary is a collection of keys and values. Keys are used to store and look up specific values within the container. This container type is named after the word dictionary, where you can look up the definition of a word. In a real-life example, the word would be the key and the definition would be the value. As an example, we can define a dictionary of television shows organized by their genre:
var showsByGenre = [ "Comedy": "Modern Family", "Drama": "Breaking Bad", ]
A dictionary looks similar to an array, but each key and value is separated by a colon (:
) here. Note that Swift is pretty forgiving with how the whitespace is used. The array could be defined with each element on its own line and the dictionary could be defined with every element on a single line. It is up to you to use whitespace to make your code as readable as possible.
With the dictionary defined previously, you would get the value "Modern Family"
if you looked up the key "Comedy"
. In code, you can access a value similar to an array, but instead of providing an index in the square brackets, you provide the key:
showsByGenre["Comedy"] // Modern Family
You can define an empty dictionary similar to an empty array, but with a dictionary, you must also include a colon in-between the brackets: [:
].
Adding a value to a dictionary looks similar to retrieving a value, but you use the assignment operator (=
):
showsByGenre["Variety"] = "The Colbert Report"
As a bonus, this can also be used to change the value of an existing key.
You might have noticed that all of my variable and constant names begin with a lowercase letter, and each subsequent word starts with a capital letter. This is called Camel case and it is the widely accepted way to write variable and constant names. The use of this technique will make it easier for other programmers to understand your code.
Now that we know about Swift's basic containers, let's explore what they are in a little more detail.