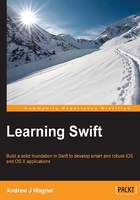
Extensions
Up until this point, we had to define our entire custom type in a single file. However, it can sometimes be useful to separate out part of our custom types in different files or even just within the same file. For this, Swift provides a feature called extensions. Extensions allow us to add additional functionality to the existing types from anywhere.
This functionality is limited to additional functions and additional computed properties:
extension Building { var report: String { return "This building is \(self.squareFootage) square feet" } func isLargerThanOtherBuilding(building: Building) -> Bool { return self.squareFootage > building.squareFootage } }
Note that to define an extension, we use the extension
keyword followed by the type we would like to extend. This can be used on any class, struct, or enumeration, even those defined within Swift, such as String
. Let's add an extension to String
, which allows us to repeat a string any number of times:
extension String { func repeat(nTimes: Int) -> String { var output = "" for index in 0..<nTimes { output += self } return output } } "-".repeat(4) // ----
This is just one simple idea, but it can often be incredibly useful to extend the built-in types.
Now that we have a good overview of what tools we have at our disposal to organize our code, it is time to discuss an important concept in programming called the scope.