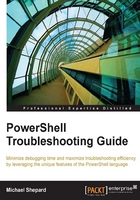
Modules
In Version 1.0 of PowerShell, the only ways to group lists of functions were to either put script files for each function in a directory or to include several functions in a script file and use dot-sourcing to load the functions. Neither solution provided much in the way of functionality, though. Version 2.0 introduced the concept of modules. A PowerShell module usually consists of a folder residing in one of the directories listed in the PSModulePath
environment variable and contains one of the following:
- A module file (
.psm1
) with the same name as the folder - A module manifest (
.psd1
) with the same name as the folder - A compiled assembly (
.dll
) with the same name as the folder
One tremendous advantage that modules have over scripts is that while every function in a script is visible when the script is run, visibility of functions (as well as variables and aliases) defined within a module can be controlled by using the Export-ModuleMember
cmdlet.
The following module file, named TroubleShooting.psm1
, re-implements the Get-PowerShellMessage
function from earlier in the chapter using a helper function (Get-Message
). Since only Get-PowerShellVersionMessage
was exported, the helper function is not available after the module is imported but it is available to be called by the exported function.
function Get-Message{ param($ver,$name) return "We're using $ver, $name!" } function Get-PowerShellVersionMessage{ param($name) $version=$PSVersionTable.PSVersion $message=Get-Message $version $name return $message } Export-ModuleMember Get-PowerShellVersionMessage
Importing a module is accomplished by using the Import-Module
cmdlet. Version 3.0 of PowerShell introduced the concept of automatic importing. With this feature enabled, if you refer to a cmdlet or function that does not exist, the shell looks in all of the modules that exist on the system for a matching name. If it finds one, it imports the module automatically. This even works with tab-completion. If you hit the Tab key, PowerShell will look for a cmdlet or function in memory that matches, but If it doesn't find one it will attempt to load the first module that has a function whose name matches the string you're trying to complete. Listing the cmdlets that have been loaded by a particular module is as simple as the Get-Command
–Module
module name.