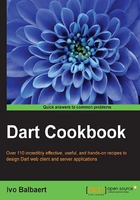
Microtesting your code with assert
Writing tests for your app is necessary, but it is not productive to spend much time on trivial tests. An often underestimated Dart keyword is assert, which can be used to test conditions in your code.
How to do it...
Look at the code file microtest.dart
, where microtest
is an internal package as seen in the previous recipe:
import 'package:microtest/microtest.dart'; void main() { Person p1 = new Person("Jim Greenfield", 178, 86.0); print('${p1.name} weighs ${p1.weight}' ); // lots of other code and method calls // p1 = null; // working again with p1: assert(p1 is Person); p1.weight = 100.0; print('${p1.name} now weighs ${p1.weight}' ); }
We import the microtest
library, which contains the definition of the Person
class. In main()
, we create a Person
object p1
, go through lots of code, and then want to work with p1
again, possibly in a different method of another class. How do we know that p1
still references a Person
object? In the previous snippet, it is obvious, but it can be more difficult. If p1
was, for example, dereferenced, without assert we would get the exception NoSuchMethodError: method not found: 'weight='.
However, if we use the assert statement, we get a much clearer message: AssertionError: Failed assertion: line 9 pos 9: 'p1 is Person' is not true. You can test it by uncommenting the line p1 = null
.
How it works...
The assert
parameter is a logical condition or any expression (such as calling a function returning a Boolean value) that resolves to false or true. If its value is false, the normal execution is stopped by throwing an AssertionError
.
Use assert
to test any non-obvious conditions in your code; it can replace a lot of simple unit tests or unit tests that can be difficult to set up. Rest assured assert
only works in the checked mode; it does not affect the performance of your deployed app because it is ignored in the production mode.
There's more...
Testing with assert
is often very useful when entering a method to test conditions on parameters (preconditions), and on leaving a method testing the return value (postconditions). You can also call a test
function (which has to return a Boolean value) from assert
such as assert(testfun
ction());
.