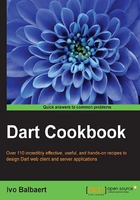
What this book covers
Chapter 1, Working with Dart Tools, talks about increasing your mastery of the Dart tools and platform. We discuss some of the more advanced and hidden features of the Dart Editor, such as configuration, compilation to JavaScript, and the pub package manager. When relevant, we also take a look at how to perform tasks with command-line tools.
Chapter 2, Structuring, Testing, and Deploying an Application, focuses mainly on all the different tasks in the life cycle of your project that make it more professional, helping you save a lot of time during the maintenance phase. This includes structuring the project and installing a logging tool in it and then testing, documenting, profiling, and publishing it.
Chapter 3, Working with Data Types, is about working with the different data types Dart has to offer. We will talk about the basic data types as well as strings, random numbers, complex numbers, dates and times, enums, and lists. Along the way, we will cover many tricks that will help you out in specific circumstances.
Chapter 4, Object Orientation, delves deeper into the object-oriented nature of Dart to find some new techniques and insights that will help us to be more productive in building our apps.
Chapter 5, Handling Web Applications, covers a wide range of web-related topics dealing with safety, browser storage, caching, event handling, WebGL, and of course, Dart working together with JavaScript.
Chapter 6, Working with Files and Streams, shows you how to work with files in different circumstances, both in synchronous and asynchronous ways. We will delve into the code to download a file both on a web and server clients, with blobs as a special case. We also discuss how transforming a stream works.
Chapter 7, Working with Web Servers, looks at how you can write full-fledged and performant web servers in Dart, more specifically how to receive data on the server, how to serve files, and how to deploy a web service. Sockets and their secure variants, as well as web sockets, are also discussed.
Chapter 8, Working with Futures, Tasks, and Isolates, concentrates on the asynchronous tools in Dart to write elegant code in future and combine their possibilities with the execution of tasks and isolates to enhance the concurrency of our apps.
Chapter 9, Working with Databases, explains how to store data in databases, on the client or server or both. On the client side, we look at IndexedDB and the Lawndart data manager. Then, we investigate how to store data on the server in SQL as well as NoSQL database systems.
Chapter 10, Polymer Dart Recipes, shows how to use Polymer to modularize the way a web client interface is built by using web components that encapsulate structure, style, and behavior. The structure and style come from a combination of HTML5 and CSS with special extensions that enable two-way data binding. Behavior is described by code contained in a class that hooks up with the component.
Chapter 11, Working with Angular Dart, covers how Angular makes it possible to write web-based apps with Model-View-Controller (MVC) capabilities in order to make both development and testing easier. The templating system is discussed along with controllers, components, views, formatters, and services.