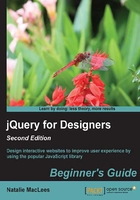
Time for action – simple custom tooltips
We'll start off by creating a simple replacement for the browser's default tooltips that we can style any way we'd like. Perform the following steps:
- Set up a basic HTML file and associated files and folders like we did in the
title
attributes as follows:<div class="content"> <h2 id="pb-gallery">Photo Gallery</h2> <ul class="gallery"> <li><img src="images/bridge.jpg" title="One of many bridges in Pittsburgh"/></li> <li><img src="images/downtown.jpg" title="Downtown Pittsburgh with bridges"/></li> <li><img src="images/icecream.jpg" title="A great way to beat the summer heat"/></li> </ul> </div>
Feel free to use CSS to style this list in the way like. If you open the page in a browser and move your mouse over the images, you'll see the text that's contained in the
title
attributes displayed as tooltips. Where the tooltip appears and what it looks like will depend on your browser, but here's how it looks in mine (Google Chrome on Mac OS): - Now, let's spruce that up a bit by replacing the default browser tooltip with our own styled one, at least for our site visitors that have JavaScript enabled. First, we'll need a copy of Steven Benner's jQuery PowerTip plugin. It's available on GitHub at http://stevenbenner.github.io/jquery-powertip/. The GitHub page has a list of features, some sample demos, the documentation you'll need to learn to use the plugin, and a link to the files available for download. Click on the green Download button to download a ZIP file that consists all the files you'll need. For this, have a look at the following screenshot:
- Unzip the file you downloaded and examine its contents. Inside, you'll find a
css
folder with several.css
files, anexamples
folder with a few working examples for you to look at, two JavaScript files, and aLICENSE.txt
file. Have a look at the following screenshot:Let's start with all the CSS files. You'll find two files named
jquery.powertip.css
andjquery.powertip.min.css
. These two files are the default tooltip styles for this plugin and have exactly the same content. The difference between them is that the second file is minified, making it smaller and ideal for use in production. The other file is a development version that we could easily edit ourselves or use as an example if we wanted to write our own custom styles for our tooltips.The rest of the CSS files are assorted styles and color schemes for the tooltips. If you look closely, you'll see the names of colors in the filenames, for example,
jquery.powertip-purple.css
orjquery.powertip-blue.css
. Each of these files also have a minified production version and a development version. All of these styles are prewritten and available to you to use in your project.You can select one of these CSS files and attach it to your page. Copy
jquery.powertip.css
to your ownstyles
folder and then attach the file to your HTML document in the<head>
section, as follows:<head> <title>Chapter 2: jQuery for Designers</title> <link rel="stylesheet" href="styles/styles.css"> <link rel="stylesheet" href="styles/jquery.powertip.css"> </head>
- Next, let's look at the JavaScript files. We have
jquery.powertip.js
andjquery.powertip.min.js
. Just like the CSS files, these are two different versions of the same file, and we simply have to choose one and attach it to our HTML document. The first file,jquery.powertip.js
, is the development version of the file and the largest at 35 KB. The second file is minified and is just 9 KB. As we don't need to edit the plugin itself and are going to use it as it is, let's select the smaller minified version. Copyjquery.powertip.min.js
to your ownscripts
folder and attach it at the bottom of your HTML file, between jQuery and your ownscripts.js
file. This is shown in the following code:<script src="scripts/jquery.js"></script> <script src="scripts/jquery.powertip.min.js"></script> <script src="scripts/scripts.js"></script> </body> </html>
- The last thing we need to do is call the plugin code. Open your
scripts.js
file and add the following document ready statement and function:$(document).ready(function(){ });
- Inside the function, select the images inside the list and call the
powerTip
method on these links, as shown in the following code:$(document).ready(function(){ $('.gallery img').powerTip(); });
Now, when you view the page in the browser and move your mouse over the images with the
title
attributes, you'll see the PowerTip-styled tooltips instead of the browser's default tooltips, as seen in the following screenshot:The default style for PowerTip tooltips is a slightly transparent black tooltip that appears directly above the item you're hovering your mouse over. These tooltips will appear with this same style, no matter which browser and operating system we're using, except that the tooltip will be opaque in browsers that don't support RGBA colors for transparency.
What just happened?
We downloaded the jQuery PowerTip plugin and attached one CSS file and one JavaScript file to our HTML document. Then, we added just a couple lines of jQuery code to activate the custom tooltips.
We selected all the images in the gallery list. We did this by taking advantage of jQuery's CSS selectors:
$('.gallery img')
Once we've selected all the images, all that was left to do was call the powerTip
method that the PowerTip plugin provided for us. The powerTip
method takes care of all the actions that need to be performed to replace the default tooltip with a custom one. But what if we want to alter the style or placement of the tooltips? Let's take a look at how we can customize the tooltips.