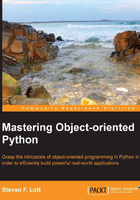
Pythonic Classes via Special Methods
Python exposes a great deal of its internal mechanisms through its special method names. The idea is pervasive throughout Python. A function such as len()
will exploit the __len__()
special method of a class.
What this means is that we have a tidy, universal public interface (len(x)
) that works on any kind of class. Python's polymorphism is based in part on the way any class can implement a __len__()
method; objects of any such class will respond to the len()
function.
When we define a class, we can (and should) include these special methods to improve the integration between our class and the rest of Python. Part 1, Pythonic Classes via Special Methods, will extend basic object-oriented programming techniques to create classes that are more Pythonic. Any class should be able to integrate seamlessly with other parts of Python. A close fit with other parts of Python will allow us to use many language and standard library features, and the clients of our packages and modules will be more confident about using them and more successful in maintaining and extending them.
In a way, our classes can appear as extensions of Python. We want our classes to be so much like native Python classes that distinctions between language, standard library, and our application are minimized.
The Python language uses a large number of special method names. They fall into the following few discrete categories:
- Attribute Access: These special methods implement what we see as
object.attribute
in an expression,object.attribute
on the left-hand side of an assignment, andobject.attribute
in adel
statement. - Callables: This special method implements what we see as a function that is applied to arguments, much like the built-in
len()
function. - Collections: These special methods implement the numerous features of collections. This involves methods such as
sequence[index]
,mapping[key]
, andsome_set|another_set
. - Numbers: These special methods provide arithmetic operators and comparison operators. We can use these methods to expand the domain of numbers that Python works with.
- Contexts: There are two special methods we'll use to implement a context manager that works with the
with
statement. - Iterators: There are special methods that define an iterator. This isn't essential since generator functions handle this feature so elegantly. However, we'll take a look at how we can design our own iterators.
A few of these special method names have been introduced in Python 3 Object Oriented Programming. We'll review these topics and introduce some additional special method names that fit into a kind of basic category.
Even within this basic category, we've got deeper topics to discover. We'll start with the truly basic special methods. There are some rather advanced special methods that are thrown into the basic category because they don't seem to belong anywhere else.
The __init__()
method permits a great deal of latitude in providing the initial values for an object. In the case of an immutable object, this is the essential definition of the instance, and clarity becomes very important. In the first chapter, we'll review the numerous design alternatives for this method.