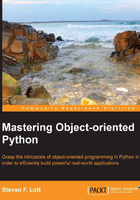
Faulty factory design and the vague else clause
Note the structure of the if
statement in the card()
function. We did not use a catch-all else
clause to do any processing; we merely raised an exception. The use of a catch-all else
clause is subject to a tiny scrap of debate.
On the one hand, it can be argued that the condition that belongs on an else
clause should never be left unstated because it may hide subtle design errors. On the other hand, some else
clause conditions are truly obvious.
It's important to avoid the vague else
clause.
Consider the following variant on this factory function definition:
def card2( rank, suit ): if rank == 1: return AceCard( 'A', suit ) elif 2 <= rank < 11: return NumberCard( str(rank), suit ) else: name = { 11: 'J', 12: 'Q', 13: 'K' }[rank] return FaceCard( name, suit )
The following is what will happen when we try to build a deck:
deck2 = [card2(rank, suit) for rank in range(13) for suit in (Club, Diamond, Heart, Spade)]
Does it work? What if the if
conditions were more complex?
Some programmers can understand this if
statement at a glance. Others will struggle to determine if all of the cases are properly exclusive.
For advanced Python programming, we should not leave it to the reader to deduce the conditions that apply to an else
clause. Either the condition should be obvious to the newest of n00bz, or it should be explicit.