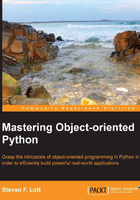
Initialization with type validation
Type validation is rarely a sensible requirement. In a way, this might be a failure to fully understand Python. The notional objective is to validate that all of the arguments are of a proper type. The issue with trying to do this is that the definition of proper is often far too narrow to be truly useful.
This is different from validating that objects meet other criteria. Numeric range checking, for example, may be essential to prevent infinite loops.
What can create problems is trying to do something like the following in an __init__()
method:
class ValidPlayer: def __init__( self, table, bet_strategy, game_strategy ): assert isinstance( table, Table ) assert isinstance( bet_strategy, BettingStrategy ) assert isinstance( game_strategy, GameStrategy ) self.bet_strategy = bet_strategy self.game_strategy = game_strategy self.table= table
The isinstance()
method checks circumvent Python's normal duck typing.
We write a casino game simulation in order to experiment with endless variations on GameStrategy
. These are so simple (merely four methods) that there's little real benefit from inheritance from the superclass. We could define the classes independently, lacking an overall superclass.
The initialization error-checking shown in this example would force us to create subclasses merely to pass the error check. No usable code is inherited from the abstract superclass.
One of the biggest duck typing issues surrounds numeric types. Different numeric types will work in different contexts. Attempts to validate the types of arguments may prevent a perfectly sensible numeric type from working properly. When attempting validation, we have the following two choices in Python:
- We write validation so that a relatively narrow collection of types is permitted, and someday the code will break because a new type that would have worked sensibly is prohibited
- We eschew validation so that a broad collection of types is permitted, and someday the code will break because a type that would not work sensibly was used
Note that both are essentially the same. The code could perhaps break someday. It either breaks because a type was prevented from being used even though it's sensible or a type that's not really sensible was used.