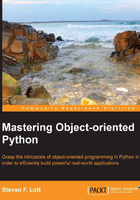
The __repr__() and __str__() methods
Python has two string representations of an object. These are closely aligned with the built-in functions repr()
, str()
, print()
, and the string.format()
method.
- Generally, the
str()
method representation of an object is commonly expected to be more friendly to humans. This is built by an object's__str__()
method. - The
repr()
method representation is often going to be more technical, perhaps even a complete Python expression to rebuild the object. The documentation says:For many types, this function makes an attempt to return a string that would yield an object with the same value when passed to
eval()
.This is built by an object's
__repr__()
method. - The
print()
function will usestr()
to prepare an object for printing. - The
format()
method of a string can also access these methods. When we use{!r}
or{!s}
formatting, we're requesting__repr__()
or__str__()
, respectively.
Let's look at the default implementations first.
The following is a simple class hierarchy:
class Card: insure= False def __init__( self, rank, suit ): self.suit= suit self.rank= rank self.hard, self.soft = self._points() class NumberCard( Card ): def _points( self ): return int(self.rank), int(self.rank)
We've defined two simple classes with four attributes in each class.
The following is an interaction with an object of one of these classes:
>>> x=NumberCard( '2', '♣') >>> str(x) '<__main__.NumberCard object at 0x1013ea610>' >>> repr(x) '<__main__.NumberCard object at 0x1013ea610>' >>> print(x) <__main__.NumberCard object at 0x1013ea610>
We can see from this output that the default implementations of __str__()
and __repr__()
are not very informative.
There are two broad design cases that we consider when overriding __str__()
and __repr__()
:
- Non-collection objects: A "simple" object doesn't contain a collection of other objects and generally doesn't involve very complex formatting of that collection
- Collection objects: An object that contains a collection involves somewhat more complex formatting