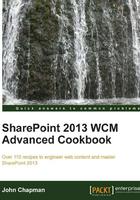
Using PowerShell to apply master page and logo settings to all sites in a farm
For this recipe, we are using a PowerShell script to apply master page and logo settings to each SharePoint site in every site collection of each web application on the local SharePoint farm.
How to do it...
Follow these steps to apply master page and logo settings to all sites in the local SharePoint farm using PowerShell:
- Open your preferred text editor to create the
.ps1
script file. - Use a
foreach
loop to iterate through each content ofSPWebApplication
on the local SharePoint farm using theGet-SPWebApplication
Cmdlet:foreach($webApp in (Get-SPWebApplication))
- Use a
foreach
loop to iterate through eachSPSite
in theSites
property of theSPWebApplication
object:foreach($site in $webApp.Sites)
- Verify the
CompatibilityLevel
property ofSPSite
to ensure it is in SharePoint 2013 (Version 15) mode and not in SharePoint 2010 (Version 14) mode.if ($site.CompatibilityLevel –eq 15)
- Use a
foreach
loop to iterate through eachSPWeb
in theAllWebs
property of theSPSite
object:foreach ($web in $site.AllWebs)
- Check if the
SPWeb
object exists:if ($web.Exists)
- Set the master page and logo properties for the
SPWeb
object:$web.SiteLogoUrl = "/SiteAssets/logo.png" $web.SiteLogoDescription = "My PowerShell Site" $web.MasterUrl = "/_catalogs/masterpages/seattle.master" $web.CustomMasterUrl = "/_catalogs/masterpages/seattle.master"
- Use the
Update
method on theSPWeb
object to save the changes:$web.Update()
- Use the
Dispose
method to discard theSPWeb
object:$web.Dispose()
- Use the
Dispose
method to discard theSPSite
object:$site.Dispose()
- Save the file as a
PS1
file, for example,applymasterpageandlogo.ps1
. - Execute the script in the PowerShell session:
./applymasterpageandlogo.ps1
How it works...
In this recipe, we retrieved all of the content web applications using the Get-SPWebApplication
Cmdlet. We then iterated through each site collection in the Sites
property of each web application and then iterated through each site in the AllWebs
property of each site collection. For each site, we updated the properties for the logo and master pages.
There's more...
The steps performed in PowerShell may also be completed in code using the server-side object model. Follow these steps to apply master page and logo settings to all sites on the local SharePoint farm with code using the server-side object model:
- Use a
foreach
loop to iterate through each contentSPWebApplication
on the local SharePoint farm:foreach (var webApp in SPWebService.ContentService.WebApplications)
- Use a
foreach
loop to iterate through eachSPSite
in theSites
property of theSPWebApplication
object:foreach (var site in webApp.Sites)
- Verify the
CompatibilityLevel
property ofSPSite
to ensure it is in SharePoint 2013 (Version 15) mode and not in SharePoint 2010 (Version 14) mode:if (site.CompatibilityLevel == 15)
- Use a
foreach
loop for iterating through eachSPWeb
in theAllWebs
property of theSPSite
object:foreach (var web in site.AllWebs)
- Check if the
SPWeb
exists:if (web.Exists)
- Set the master page and logo properties on the
SPWeb
object:web.SiteLogoUrl = "/SiteAssets/logo.png"; web.SiteLogoDescription = "My Code Site"; web.MasterUrl = "/_catalogs/masterpages/seattle.master"; web.CustomMasterUrl = "/_catalogs/masterpages/seattle.master";
- Use the
Update
method on theSPWeb
object to save the changes:web.Update();
- Use the
Dispose
method to discard theSPSite
andSPWeb
objects:web.Dispose(); site.Dispose();
See also
- The SPWebApplication class topic on MSDN at http://msdn.microsoft.com/en-us/library/microsoft.sharepoint.administration.spwebapplication.aspx
- The SPWeb class topic on MSDN at http://msdn.microsoft.com/en-us/library/Microsoft.SharePoint.SPWeb.aspx
- The SPSite class topic on MSDN at http://msdn.microsoft.com/en-us/library/microsoft.sharepoint.spsite.aspx
- The Get-SPWebApplication topic on TechNet at http://technet.microsoft.com/en-us/library/ff607562.aspx